Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial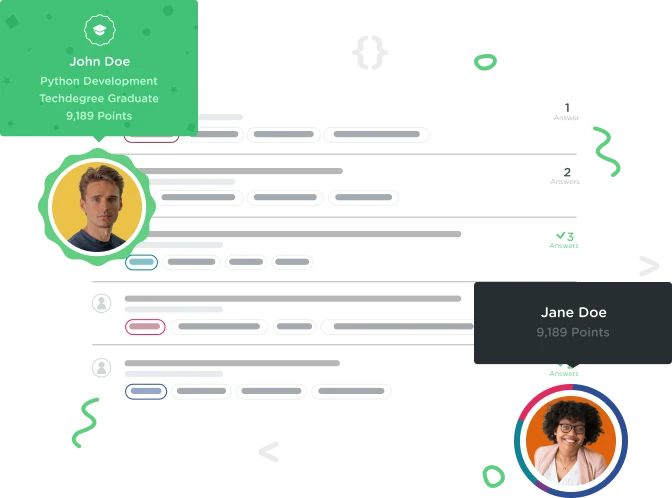

Christine Mackay
3,477 PointsI didn't add anything to the arrays, but my quiz still works fine. Is my answer okay?
To built the lists of correct and incorrect questions, I didn't add to the array as shown in this video, but rather I just used concatenation. It works just fine, but is the solution in the video better? I don't really understand the solution in the video. Please view my workspace below.
1 Answer
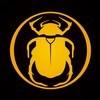
rydavim
18,814 PointsThere's nothing wrong about your solution, per se. There are always going to be multiple ways to solve any programming problem. In this case, I would say the only reason why the given solution might be preferred is that it does a better job at teaching the particular topics covered in this lesson.
Since this section is chiefly about arrays in JavaScript, I would look over the teacher's solution and review any areas you're not 100% confident about. Here's a commented version of the solution:
// Setup the questions. Note that these all have int answers.
var questions = [
['How many states are in the United States?', 50],
['How many continents are there?', 7],
['How many legs does an insect have?', 6]
];
var correctAnswers = 0; // How many correct answers.
// The next three variables aren't strictly necessary, they're more for readability.
var question; // Current question.
var answer; // Current correct answer.
var response; // Current user response.
var correct = []; // Array for correct questions.
var wrong = []; // Array for incorrect questions.
// This is the same as what you're using.
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
// This builds out a block of HTML using the given array.
// At the end, he passes in the correct and wrong arrays created above.
function buildList(arr) {
var listHTML = '<ol>'; // Create an ordered list for the type of answers (correct/wrong).
// For each answer in the current array passed, build a list item.
for (var i = 0; i < arr.length; i += 1) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ol>';
return listHTML; // Return the HTML block.
}
for (var i = 0; i < questions.length; i += 1) {
// These are the variables created for readability. The way you did it is okay too.
question = questions[i][0];
answer = questions[i][1];
response = prompt(question);
// You *do not* need this part.
// Your toLowerCase() is the appropriate way to parse *your* questions.
response = parseInt(response); // They have int-based questions.
if (response === answer) {
correctAnswers += 1; // ++ is just as good as doing it this way.
correct.push(question); // They're using push(), because they're storing it in an array.
} else {
wrong.push(question); // push() here as well, for the same reason.
}
}
html = "You got " + correctAnswers + " question(s) right." // Pretty much the same.
// Here is where their HTML gets built. This calls the buildList function created above.
html += '<h2>You got these questions correct:</h2>';
html += buildList(correct); // Passes in the created correct answers array.
html += '<h2>You got these questions wrong:</h2>';
html += buildList(wrong); // Passes in the created wrong answers array.
print(html);
Let me know if you still have any questions, but you did a nice job writing your solution. It's always good practice to try multiple ways of solving the same problem. Happy coding!
Christine Mackay
3,477 PointsChristine Mackay
3,477 PointsThank you! I think I get it now :-)