Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial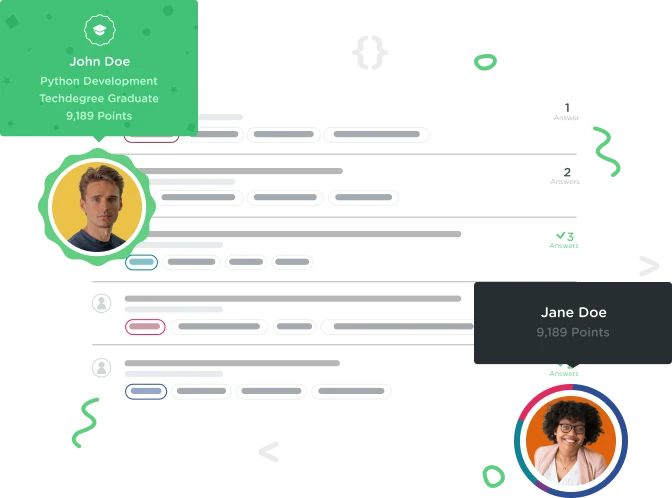

Brian Uribe
3,488 PointsI didn't fully understand the video
I'm confused on this challenge task. I'm not 100% sure what i'm doing. I know I keep asking questions, but someone please help, much appreciated.
for number in 1...10 {
println("/(number) times 7 is / (number*")
}
1 Answer
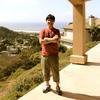
Richard Lu
20,185 PointsHey Brian,
Let's take this problem step at a time.
The challenge wants you to print out the multiples of 7. So something like this:
1 * 7
2 * 7
3 * 7
// and so on
In order to achieve the left operand (1-10), we'll use a for-in loop.
for i in 1...10 {
// loops from 1 to 10 and stores each iteration onto i. Just what we need!
}
The last step is to actually do the multiplying and printing.
for i in 1...10 { // use the loop from before
let multiple = i * 7 // multiple now contains a multiple of 7
println("\(i) * 7 = \(multiple)") // using "string interpolation", we can encapsulate other objects onto our string.
}
If you have any other questions, feel free to ask! It's a learning environment :)
- Rich
Brian Uribe
3,488 PointsBrian Uribe
3,488 PointsHello!
I'm sorry but i'm still struggling to understand. The video that goes along with this was fairly vague, I wasn't sure why they were doing what they were doing. Can you break down the last part? I understand that "i" represent any number from 1-10, and when we say i * 7, we are saying all numbers from 1-10 will be multiplied by 7, but the last part, the ("(i) * 7 = (multiple)". Also, I keep getting this challenge question wrong. Can you give me the answer and give me a break down of why that answer is correct?
Richard Lu
20,185 PointsRichard Lu
20,185 PointsHey Brian,
You're answer is pretty close. Here's the answer:
To break down the last part (string interpolation), let say we have the strings "hello" and "world". In order to combine the two strings together, you have the following:
There are different ways of accomplishing something when you program. Another way to combine the two strings is a method called string interpolation. Here's an example:
In the challenge, they want you to have an output of the following format
In order to accomplish that, we'll use "blank lines"/string interpolation
\()
.println("\(i) * 7 = \(i*7)")
Let me know if there are any additional questions. :)