Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial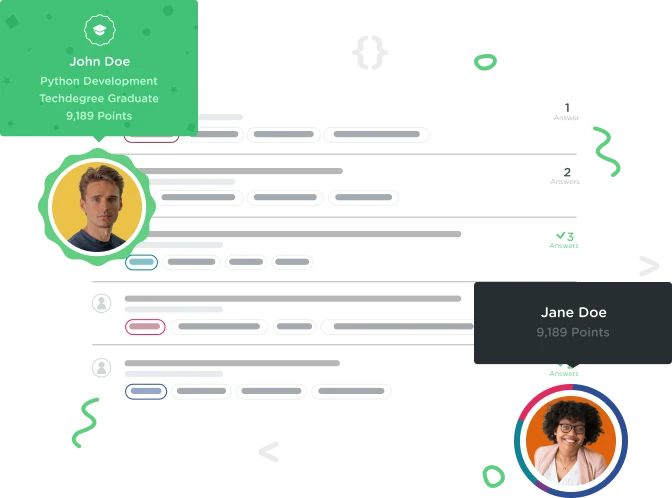

Callum Walsh
Courses Plus Student 1,453 PointsI didn't fully understand what I was meant to do. Can someone review my code?
I just want to know if my code below would be considered good or bad programming, and if bad, how could I improve it?
It does work fine however.
var questions = [
["Which animals fur pattern would you desbribe as \"Zebra Strips\".", "zebra"],
["Is the earth round?", "yes"],
["What is 10 + 10.", "20"]
];
var total = 0;
function print(message) {
for (var i = 0; i < message.length; i++) {
var answer = prompt(message[i][0]);
answer = answer.toLowerCase();
if (questions[i].indexOf(answer) > -1) {
document.write("Correct " + answer + " is the right answer to question " + (i + 1) + ", " + questions[i][0] + "<br>");
total++;
} else {
document.write("Incorrect " + answer + " is the wrong answer for question " + (i + 1) + ", the answer was " + questions[i][1] + "." + "<br>");
}
}
document.write("<br>You scored " + total + " points, out of " + questions.length);
}
print(questions);
2 Answers
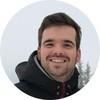
John Lack-Wilson
8,181 PointsA few points to make:
- It could be the way it is formatted on this page, but your indentation is inconsistent
- You could set the answer variable outside of the for loop (i.e. var answer = null;)
- You can chain the answer variable to reduce the amount of lines in the for loop (answer = prompt(message[i][0].toLowerCase())
- More comments to explain what the for loop is doing and also what the if statement is for - it's a little hard to understand, and you may not remember why you've done it that way later down the line
Those are just my observations, some others may have better analysis. But I hope those pointers help.
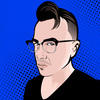
Tyler Durden
2,406 Points // 2d array of stupid questions
var questions = [ ['What is the color of the sky?', 'BLUE'],['What is a lizard?', 'REPTILE'], ['What is a dog?', 'MAMMAL']];
// counts questions answered correct; starts at 0
var correct = 0;
// stores prompt response in a variable
var ans = "";
// stores string of questions answered correct
var questionsCorrect = "";
// stores string of questions answered wrong
var questionsWrong = "";
// for loop to cycle through 2d array
for(var i=0; i<questions.length; i++) {
ans = prompt(questions[i][0]);
// if statement checks prompt response in stored in variable ans to see if true
// if it is true, then it adds +1 to var correct and stores the actual question answered correct in a string the gets concatenated for every question answered correct
if(ans.toUpperCase() == questions[i][1]) {
questionsCorrect += i+1 + '. ' + questions[i][0] + '<br>';
correct++;
}
// similiar concatenation of string as above, but this time for questions answered wrong. No added points to var correct
else {
questionsWrong += i+1 + '. ' + questions[i][0];
}
}
// tedious print statements everyone hates
document.write('You got ' + correct + ' question(s) correct' + '<p>');
document.write('You got these questions correct:' + '<br>' + questionsCorrect + '<p>');
document.write('You got these questions wrong:' + '<br>' + questionsWrong + '<p>');
Callum Walsh
Courses Plus Student 1,453 PointsCallum Walsh
Courses Plus Student 1,453 PointsThank you! Those are some good pointers that slipped my mind.