Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial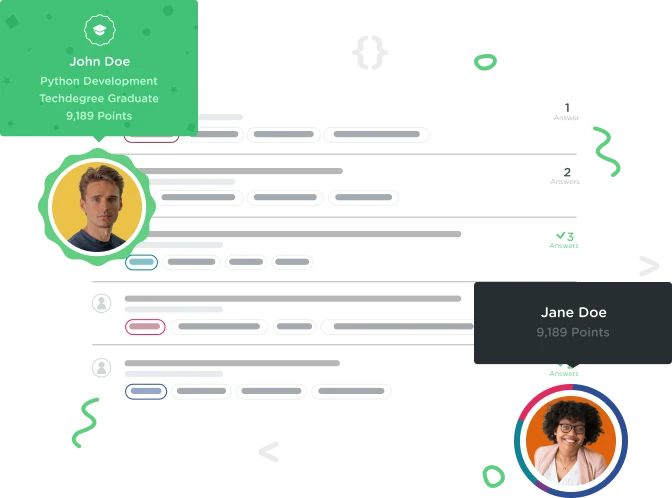

Mozart Liessi
2,618 PointsI didn't understand when Graig wrote "String veggie: vegetables"
maybe it's a very simple question, but I didn't understand it yet
3 Answers
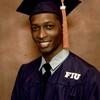
Dane Parchment
Treehouse Moderator 11,077 PointsThe colon is used in multiple places in Java, however, the most common place (in my opinion) is in the for-each loop.
Basically the for-each loop looks like this:
for(VariableType variableName: array|arrayList) {
//.......do stuff here
}
Basically what it is a loop that's sole purpose is to work for collections or lists like Array and ArrayList.
The syntax is saying: store each value indexed in the specified array or arrayList in the specified variable, then in the body of loop perform the specified action to that variable.
An example would be:
int [] list = [1, 2, 3, 4, 5, 6, 7, 8];
for(int number: list) {
System.out.println(number);
}
The output would be:
1
2
3
4
5
6
7
8
Hopefully that is enough information for you to understand how it works and what it does, if you still need help let me know.
EDIT: Remember that for the foreach loop to work the variable must be of the same type as the array, so if your array is a double then your variable should also be a double, and if it is a Dog object then your variable should also be a dog variable.

Sang Tran
6,918 PointsHey Mozart, did you mean what Craig was doing at 3:32? He's creating a For each loop/ enhanced For loop. In that example, he wants to print out each veggie in his vegetables array. The variable name veggie can be called anything btw.
Example: for (String v : vegetables) { System.out.println(v); }

Aaron Arkie
5,345 PointsHello Mozart, Craig is creating a "for-each" loop. This for-each loop is great when dealing with sifting through the elements of an array. The Syntax is as follows...
for( datatype <variable-name> : <declared-array-variable>)
{
// do something extraordinary
}
The datatype for the variable you are using to go into the array must match the elements found in the array. The best way to remember this loop is to say " For each time <variable-name> goes into my <array-variable>... do something.
You can achieve the same process using a normal for-loop
for(int veggie = 0; veggie < vegetables.length; veggie++)
{
System.out.println(vegetables[veggie]);
}
I hope this helps! This stackoverflow(http://stackoverflow.com/questions/85190/how-does-the-java-for-each-loop-work) might be of use to you.
EDIT: Changed to an answer instead of a comment - Dane E. Parchment Jr. (Moderator)
Mozart Liessi
2,618 PointsMozart Liessi
2,618 Pointsthanks very much, it got very clearly to me now