Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial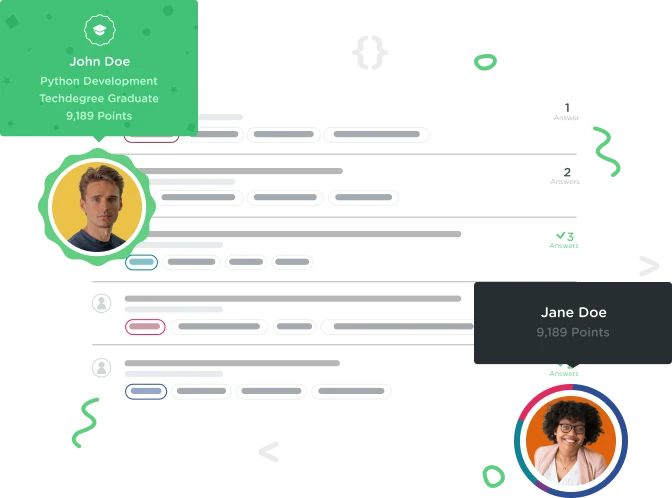

Wil Smith
1,283 PointsI do not get how this works.
I have no idea how this works. No idea how we can add 'prop' or 'key' if its not assigned to anything and it still works. Do not understand the brackets when calling the property value. My brain is bulldozed.
2 Answers
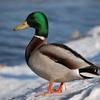
Robert Schaap
19,836 PointsTake a break :)
Any object consists of what we call key/value pairs. In the object below, the keys are "firstName" and "lastName" and their respective values are "Wil" and "Smith"
var yourObject = {
firstName: "Wil",
lastName: "Smith"
}
A for in
loop lets you loop over these. Below and in the video the word key is used, but it's essentially just a variable you've just declared. You could also write var item in yourObject
and console.log item
and it'd still work.
for (var key in yourObject) {
console.log(key)
// logs "firstName" and "lastName"
}
The above just logs the keys, that's where the brackets come in. On a regular object, if you do something like: console.log(yourObject["firstName"])
, you would be accessing the "firstName" property on the object and logging it's value. You can apply the same in the loop:
for (var key in yourObject) {
console.log(yourObject[key])
// logs "Wil" and "Smith"
}
Since your loop is already returning the key each time it runs, you can just say, get me yourObject and console.log the value of the current key.
I put the above in a jsfiddle, run it and have a look in the console. https://jsfiddle.net/u9c7p1un/6/

Yuri Timochkin
347 PointsWhy here, there is no - " " in key, like :
console.log(yourObject["firstName"]) but in loop we can use it without " " - like console.log(yourObject[firstName])?
for (var key in yourObject) { console.log(yourObject[key]) <- But in this case syntax should be console.log(yourObject["key"])
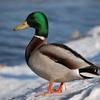
Robert Schaap
19,836 PointsObject keys are essentially strings in JavaScript. So the first time the loop runs, var
is essentially set to var key = "firstName"
and you're just passing the variable to access the value. So in that first loop yourObject[key]
is the same as yourObject["key"]
mohamed shimax
5,303 Pointsmohamed shimax
5,303 PointsHi,
For my understanding in general you always use dot notation to excess values in object literals. But if the property or key is a variable or the key wrapped inside two double quotes, Then use [] brackets to access the value.
Note that by default in object literals key is a string value unless it's not a variable.