Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial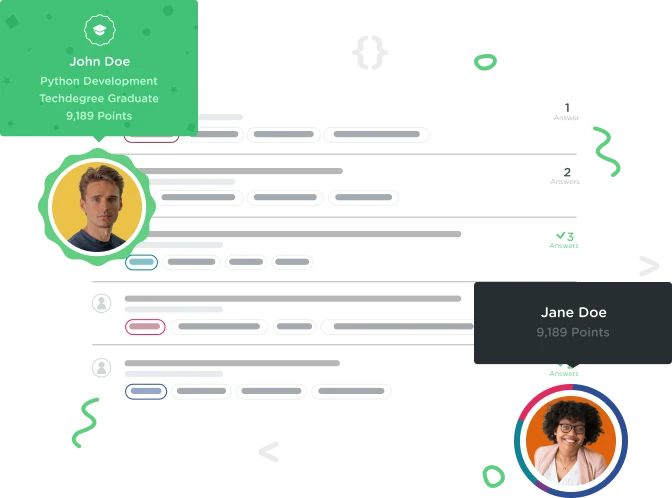

Liam Tulit
2,973 PointsI do not get the task?
I don't get the location stuff.
struct Location {
let latitude: Double
let longitude: Double
}
2 Answers
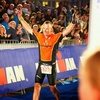
Steve Hunter
57,712 PointsHi Liam,
There's a few parts to this challenge.
First you need to create a new class called Business
- that has two stored properties; a name (of type String
) and a location (of type Location
). The Location
struct is provided for us. |Both stored properties are constant, so they're declared with let
not var
.So, let's write the class:
class Business{
let name:String
let location: Location
}
The class needs an init
method. This takes two parameters, the Location
object and the String
. These go into the init
method:
init(name: String, location: Location){
// todo
}
Inside init
we set the properties of the class to the parameters passed in, so the name
variable inside the class (self
) is assigned the value held by the parameter that was just passed in:
init(name: String, location: Location){
self.name = name
self.location = location
}
Put the init
method inside the class and that's the class complete. We now need to create an instance of it called someBusiness
. That's a constant too, so declared with let
. We then assign a Business
object to that, and pass the parameters in, as defined in the init
method. That needs a name and a Location
. A Location
object needs creating, though. That holds two stored properties, a longitude
and a latitude
- both are of type Double
so they can handle decimal points; not like an integer. We can create that like this:
Location(latitude: 53.2, longitude: 3.1)
That's one parameter sorted. The other is pretty simple, as it is a string. The whole init
method header could look like:
Business(name: "A Business", location: Location(latitude: 53.1, longitude: 3.1))
That needs assigning to someBusiness
:
let someBusiness = Business(name: "A Business", location: Location(latitude: 53.1, longitude: 3.1))
Put that lot together, and you're done!
Let me know how you get on.
Steve.
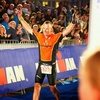
Steve Hunter
57,712 PointsHi Liam,
You need to create the instance of the class outside its definition. Also, you've declared a Location
object inside the constructor(init
) that's doing nothing - you can delete that. And there's a bracket missing at the end of your instance-creation. That does throw an error in Xcode.
So, you need to clean up your init
method (remove the redundant Location
object), then move your someBusiness
declaration outside of the class and add a bracket to it.
That should do it.
Steve.

Liam Tulit
2,973 Pointsok thanks a lot Steve!!!! :)
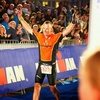
Steve Hunter
57,712 PointsNo problem - let me know how you get on.
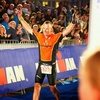
Steve Hunter
57,712 PointsThe error output from your code isn't very illuminating, it has to be said!
swift_lint.swift:17:99: error: expected ',' separator
let someBusiness = Business(name: "A Business", location: Location(latitude: 53.1, longitude: 3.1)
^
,
swift_lint.swift:18:1: error: expected expression in list of expressions
}
^
swift_lint.swift:17:99: error: expected ',' separator
let someBusiness = Business(name: "A Business", location: Location(latitude: 53.1, longitude: 3.1)
^
,
swift_lint.swift:18:1: error: expected ')' in expression list
}
^
swift_lint.swift:17:28: note: to match this opening '('
let someBusiness = Business(name: "A Business", location: Location(latitude: 53.1, longitude: 3.1)
^
swift_lint.swift:15:9: warning: result of initializer is unused
Location(latitude: 53.2, longitude: 3.1)
Only two are of any use - the one that says you've not closed the bracket and the one that says your Location
object is unused.
Liam Tulit
2,973 PointsLiam Tulit
2,973 PointsThis is my code however treehouse says there are compiler errors but Xcode runs it fine??