Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial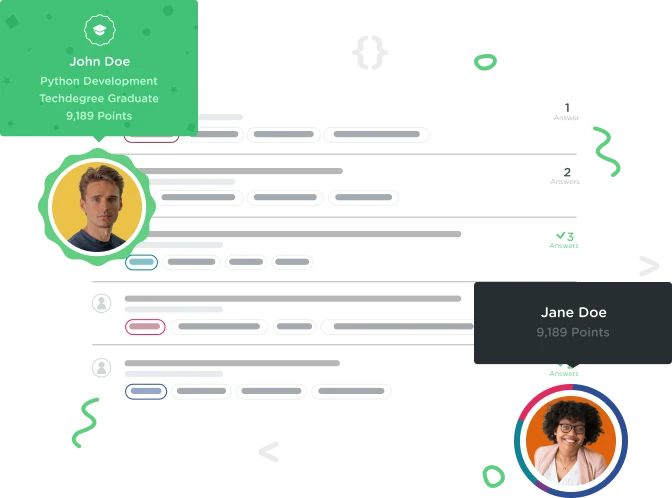

Mark Bekhet
900 PointsI do not know how to fix the problem
Now in this challenge I'm supposed to have every word in the string as a key in a dictionary and the time of the repetition as its value. My code provides me with a mistake that I understand but I don't know the way to solve it. According to this code, i'll have a list of values which is equal to the count of the word in the string. but I want only one value which is the total of repetition of the word
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
string = string.lowercase()
word = string.split()
counting = {}
word = counting.keys()
for word in string:
index = string.count(word)
index = counting.values(word)
return counting
1 Answer
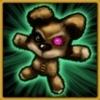
Dave Harker
Courses Plus Student 15,510 PointsHi Mark Bekhet,
Really great effort, I can see what you were trying to do there but as it stands there are a few oversights.
I thought it might help if we run through the code together as the computer would run through it. We can look at what happens to each variable and at each stage and I'll pop a thought in here or there.
After that, we'll run through one way of accomplishing the challenge goals. I hope this is helpful to you
def word_count(string):
string = string.lowercase()
# error - in Python 3 to get a string to lowercase the function is .lower()
# assuming change to lower() string is a string : 'i do not like it sam i am'
word = string.split()
# word is now a list : ['i', 'do', 'not', 'like', 'it', 'sam', 'i', 'am']
counting = {}
# counting is an empty dictionary : {}
word = counting.keys()
# word variable is now reassigned from a list of words in the string, to an empty dictionary : dict_keys([])
for word in string:
# shouldn't use variable word again (it will work because out of scope but bad practice as makes code confusing to read/maintain), but this loop will check each and every character (including spaces) in your original string(lower cased)
index = string.count(word)
# new variable index created but will never get used - in fact, it's re-initialized in the next line
# each character pass would just assign index the count of the current character in the full string
index = counting.values(word)
# error - dictionary.values() takes no arguments
# assuming no arguments passed, this will 1/ change and clear index from an Int value to an empty list as counting has no values
return counting
# counting (which is an empty dictionary) gets returned
Ok, I hope that helped some in identifying the issues. Now the good/fun stuff!! Let's work on the challenge.
I need you to make a function named word_count. It should accept a single argument which will be a string. The function needs to return a dictionary. The keys in the dictionary will be each of the words in the string, lowercased. The values will be how many times that particular word appears in the string.
def word_count(string):
# let's make a word list to hold the words from the string passed into the function
word_list = string.lower().split()
# let's remake an empty dictionary for counting the words in the word_list
counting = {}
# This loop we can use 'word' as it's singular and refers to each 'word' in our 'word_list'
for word in word_list:
# we need to check if the word is already in out counting dictionary
# if it is, we need to increment it's value by 1
if word in counting:
counting[word] += 1
# if it isn't then it's a new word.
# So let's add it to counting and set it's initial count value
else:
counting[word] = 1
# now that we've run through the word_list we made and counted the occurrences of each
# let's return the counting dictionary we've built up
return counting
There are several ways to accomplish this challenge outcome, that's just one of them.
I went through your code and explained the issues I saw in an effort to explain why it wouldn't work .. do not get disheartened, you made a really good effort and didn't just come to the forum saying 'give me the code' which is fantastic to see!
After you read this, I'd encourage you to close it and tackle the challenge again but before you code anything:
- grab some paper and a pen
- write out the full challenge
- break that down into smaller challenges
- for each challenge you break it into, think about if it can be broken down further.
- keep breaking them down, until you have a group of small, easier challenges
- work out the order you'll need to solve them in
Now, jump on the computer again and start coding!
Build up your code solving each of those small challenges.
Once they are built up and put together, you'll have the working code to solve the initial 'bigger' challenge. (which hopefully now doesn't look so big after all)
You'll probably want to refactor and make it more concise, and that's OK (in fact, it's encouraged) ... but the most important thing is breaking it down and solving it first, then worry about the 'tidy up'
Best of luck moving forward and as always ... happy coding!!
Dave
Joel Sprunger
5,448 PointsJoel Sprunger
5,448 PointsI was having trouble with this challenge. Part of the issue was that I started by removing punctuation. That must be an issue. The other problem I had was that I was using .split(" ") which doesn't remove all white spaces like .split(). Failure is the best teacher.