Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial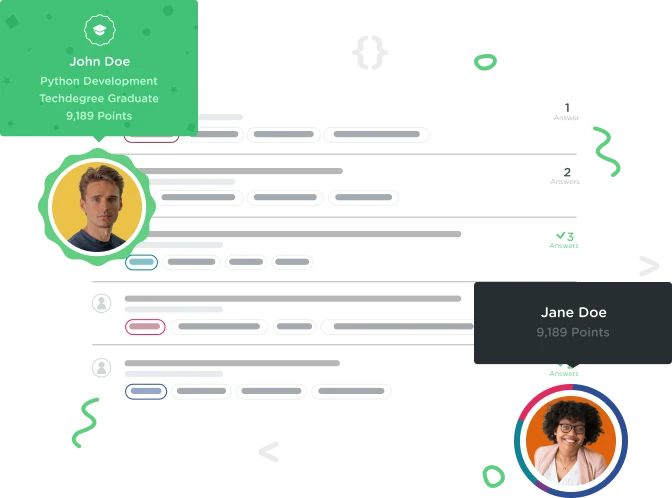
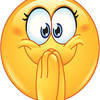
Bogdan Siverchuk
Courses Plus Student 1,707 PointsI do not know what it should return! It says it should return java.util.Set of all authors, java.util.Set is a library!
i just don't get what it should return! How can it return library?
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.Set;
import java.util.TreeSet;
import java.util.List;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public String getAllAuthors(){
Set<String> allAuthors = new TreeSet<String>();
for(BlogPost author : getPosts()){
return ????? ;
}
}
}
2 Answers

Yanuar Prakoso
15,196 PointsHi There Bogdan
Maybe what you mean is java.util.Set is an interface and to be honest you already done a good job wrapping the Set interface into TreeSet class. You even already import both packages for Set and TreeSet. However, your code in the getAllAutors method need some refinements. Most important is the method declaration. The challenge states that you need to return a Set of String consist of all authors, thus you must declare your method as: Set<String> getAllAuthors() {
The code should be like this:
public Set<String> getAllAuthors() {//<- remember they asked for a Set of String from this method
Set<String> allAuthors = new TreeSet<>();//<- wrapping up Set interface inside TreeSet class
for(BlogPost post : mPosts) {//<-- iterate each BlogPost post from the list of mPosts
allAuthors.add(post.getAuthor());//<--This is how you add authors of the posts into the Set
//Please note: post.getAuthor() is a public method in BlogPost class to get the author data of the post
}
return allAuthors;//<- we return the set... and it is all done
}
I hope this can help a little
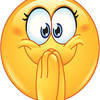
Bogdan Siverchuk
Courses Plus Student 1,707 PointsMay I ask why you do not declare TreeSet<String>? You leave <empty>

Yanuar Prakoso
15,196 PointsHi Bogdan..
In Java programming when You declare using <.....> it is called generics. It basically parameterized over types. Long story short in Java SE 7 and later, you can replace the type arguments required to invoke the constructor of a generic class with an empty set of type arguments (<>) as long as the compiler can determine, or infer, the type arguments from the context. This pair of angle brackets, <>, is informally called the diamond. For example:
Set<String> someSet = new TreeSet<String>();
Since the member variable of both sides in the new declaration is the same (<String>) you can just write:
Set<String> someSet = new TreeSet<>();
It means the same when you use map for instance:
Map<String, Video> someMap = new HashMap<String, Video>();
Since both sides have the same member variables types (String as key and Video as value) you can shorten the code:
Map<String, Video> someMap = new HashMap<>();
I hope this can help