Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial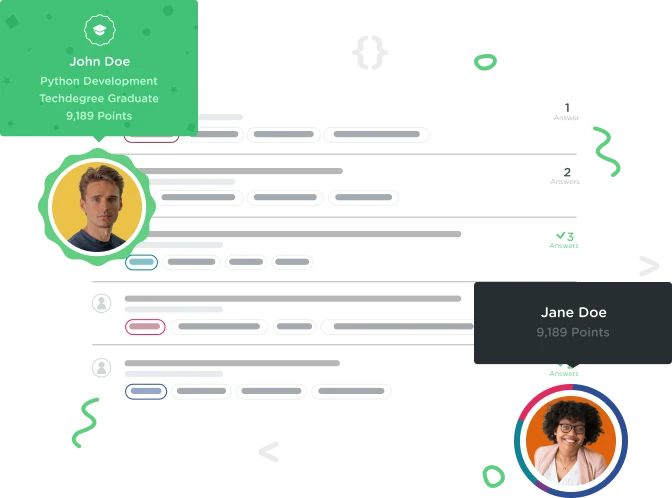

KJ Han
987 PointsI do not know what step to take next!
Ok, so the mission is to sort given last names based on their first letter. For example, if the first letter of a last name is in between A to M, it should return 1. If it is between N to Z, it should return 2.
I currently do not have enough knowledge/experience in Java to tackle this problem... Any suggestions or help to get through this problem will be much appreciated!
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
char firstLetter = lastName.charAt(0);
if (firstLetter
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
1 Answer
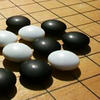
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsThe Example.java class gives you a hint: you can compare characters to find out if they are before or after each other in the alphabet. So what you need to do is get the first character of the string. Then you can compare it with the 'N' character. If it's before 'N' (smaller than 'N') the result is line 1, otherwise the result is line 2.
Have a look at the java doc of String, got the "Methods" section, and look for a method that returns a char. You should be able to find one you can use to get the first character.
KJ Han
987 PointsKJ Han
987 PointsThanks for your advice, I found it very helpful! Have a nice day :)