Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial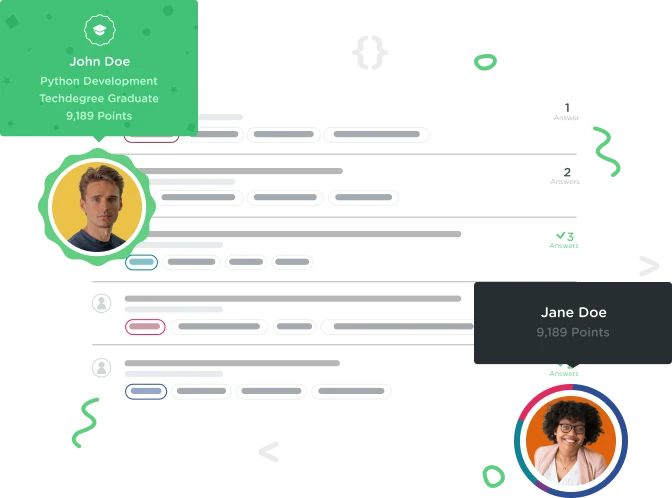
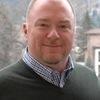
Michael Wedge
803 PointsI do not understand how to complete this Challenge. Any assistance would be appreciated.
Working on completing this challenge. I do not understand what I a missing to complete the creation of the class Square, and how to initialize sideLength to 4 using the Base() construct.
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(sideLength)
{
}
}
}
2 Answers
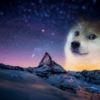
Connor Walker
17,985 PointsWe know that a square will always have 4 sides, so we send 4 to the base class to fill in the NumSides property as this is never going to change (so we don't need to pass a variable). Then all it wants you to do is assign the "SideLength" property in the square class using the constructor and the value that has been passed to it (sideLength in this case). It should look like this:
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(4)
{
SideLength = sideLength;
}
}

fodhil zidane
24,020 Pointsnamespace Treehouse.CodeChallenges { class Polygon { public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(4)
{
SideLength = sideLength;
}
}
}

fodhil zidane
24,020 PointsThis code passed correctly