Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial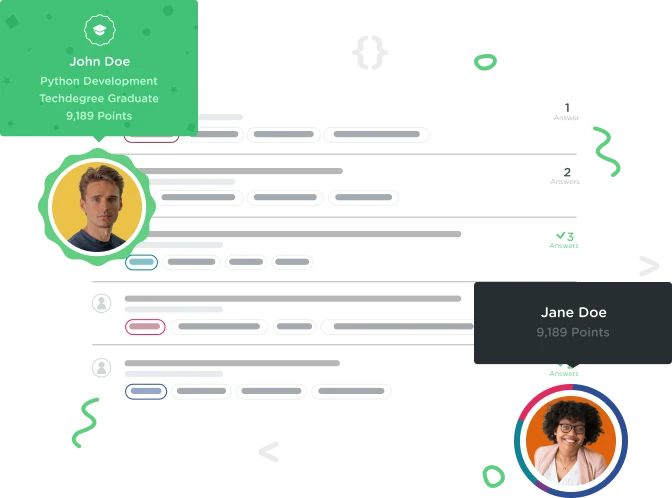

Adrian Yao
1,002 PointsI do not understand what I am doing wrong?
I know I am wrong but the logic checks out?
struct Person {
let firstName: String
let lastName: String
func fullName(ofPerson person: String = firstName + lastName) -> (String)
}
}
1 Answer
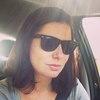
Maria Angelica Dadalt
6,197 PointsHi, I just answered a fellow student with the same doubts you have. Be sure to search for answers in the community, almost always somebody else had a problem with the same challenge at some point. I know I did!
This is the same answer I gave the other person:
So here is where you went wrong. The instruction doesn't ask for parameters in your function, but you do need to return a string. So the set up for the method should look like this:
func fullName() -> String {
}
Now you need to set up a concatenation or interpolation of the string to return the full name.
func fullName() -> String {
return firstName + " " + lastName
}
or
func fullName() -> String {
return "\(firstName) \(lastName)"
}
Now you have to create an instance of Person using a let called aPerson with values of your choice:
let aPerson = Person(firstName: "Maria Angelica", lastName: "Dadalt")
And finally, you have to call on the method (or function, whichever you prefer), using the instance you created with a dot notation.
let myFullName = aPerson.fullName()
I hope this could help you!