Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial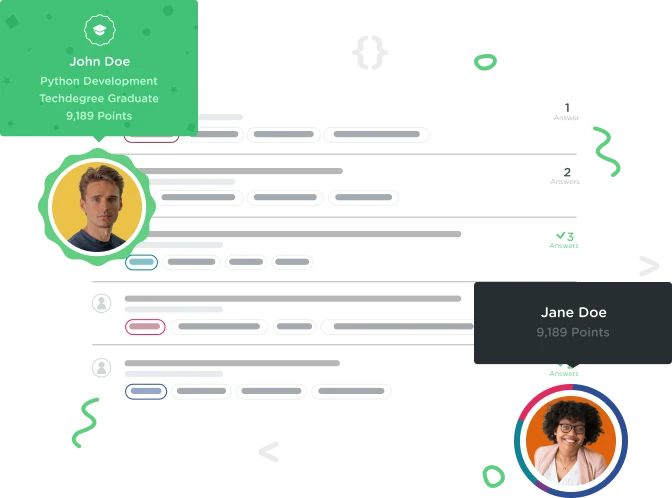

Eddie Aguilar
2,393 PointsI do not understand why i need string interpolation
Curious to know why i need to use string interpolation with the self.description statement. When i was working through everything, I thought I had written everything I needed to, but when I looked up the question on the internet, I was missing the interpolated part (below is what i did) And again, I just don't understand why we need string interpolation in the statement, can someone please explain, in detail, why?
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// THIS WOULD BE WHAT I WROTE BELOW
init(red:Double, green:Double, blue:Double, alpha:Double) {
self.red = 86.0
self.green = 191.0
self.blue = 131.0
self.alpha = 1.0
self.description
}
}
1 Answer

Martin Wildfeuer
Courses Plus Student 11,071 PointsExcerpt from the challenge: "For example, given the values 86.0 for red, 191.0 for green, 131.0 for blue and 1.0 for alpha, each of the stored properties should hold these values and the description property should look like this: "red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0"
You want description to represent the RGB values with one single String. This might come in handy for debugging, for example, as you can quickly print all colors via print(instanceOfRGBColor.description)
However, all color values and alpha are of type Double. This is where string interpolation comes in handy.
test
would combine everything to a convenient String:
// Variables of any type can be inserted in a string by enclosing them with \()
let test = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
In your code, the description
value needs to be assigned a value at initialization, this is mandatory.
So instead of having this in your initializer...
// self.description only is invalid syntax. This will only work in playgrounds
self.description
...you have to assign description
an actual value. As requested for this challenge, it has to be like the example string:
"red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0"
Therefore, it should be:
// self.description only is invalid syntax. This will only work in playgrounds
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
Also, with your code, although the initializer is correct, you are actually not using the values you are passing in. Actually, you are giving them fixed values, so whatever values you are passing the initializer, it will still always the same.
These things combined with your code:
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
// Assigning the interpolated String to description, just
// as the other values
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
I hope that makes sense :)