Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial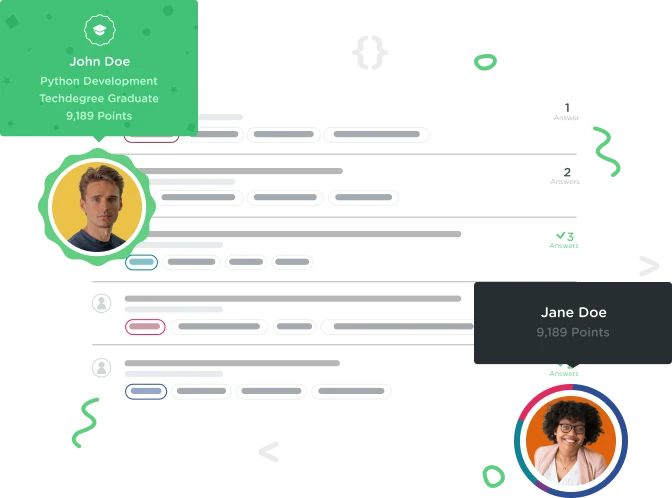

Benjamin Sumser
1,277 PointsI do not understand why my code is not being accepted by the website. It works perfectly in pycharm.
My code works perfectly when I enter "The quick brown fox jumps over the lazy dog." but the code challenge keeps rejecting it saying it is getting back unexpected letters.
Am I missing something?
def disemvowel(word):
vowels = ['a','e','i','o','u']
word2 = ''
for letter in word.lower():
if letter not in vowels:
word2 += letter
return word2
This code also works in pycharm, but is not accepted by the site:
def disemvowel(word):
word_list = list(word)
vowels = ['a','e','i','o','u']
for letter in word.lower():
if letter in vowels:
word_list.remove(letter)
word = ''.join(word_list)
return word
2 Answers
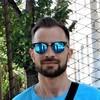
Oszkár Fehér
Treehouse Project ReviewerHi Benjamin,
Your codes are perfect, they work both, the problem is that the word it's lowercase in the for loop
for letter in word.lower():
it should be
for letter in word:
In the quiz this sentence
Oh, be sure to look for both uppercase and lowercase vowels! suggest that the input word must checked how it is. So the
vowels = ['a','e','i','o','u']
should contain
vowels = ['a','e','i','o','u','A','E','I','O','U']
I hope it helps you. Great work.

Greg Kaleka
39,021 PointsHey Benjamin,
I like the idea of checking for lowercase vowels against the lowercased word. You're right that it removes the vowels (both upper- and lowercase). The problem is that the output ends up all lowercase. So for example:
disemvowel("Hello World!")
Should return "Hll Wrld!"
, but if you lowercase word
, it will instead return "hll wrld!"
. That's not what we want - it's doing more than just removing the vowels from the original input.
As is often the case in programming, there's more than one way to solve this problem.
Oszkár Fehér's answer is one way to solve this (a good one!), but if you prefer to keep the shorter, lowercase-only list of vowels (note you actually don't need a list - you can check if a letter is in
a string), you could call .lower()
on the letter you're checking against. It would look like this:
def disemvowel(word):
word_list = list(word)
vowels = 'aeiou'
for letter in word:
if letter.lower() in vowels:
word_list.remove(letter)
word = ''.join(word_list)
return word
This has the nicer, shorter list of vowels you used, and maintains the case of the consonants in the original word.
Hope that's helpful
-Greg

Benjamin Sumser
1,277 PointsLower the local variable! That is great! Later I caught the problem of not returning the same case as entered. That is why I tried the second set of code, which did return the original cases. But it was still not allowed. Can you explain my error in the second one?
edit: Because the lowered vowel won't be found in the original list I made... Wowza. How do you see this stuff? I hope I get that proficient.

Greg Kaleka
39,021 PointsThe second one will throw an error if you have any capitalized vowels. For example, let's say your word is "Open". The loop will start with O:
#...
for letter in word.lower(): # open
if letter in vowels: # o is in vowels
word_list.remove(letter) # trying to remove "o" from ["O", "p", "e", "n"] throws an error.
#...
Make sense?