Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial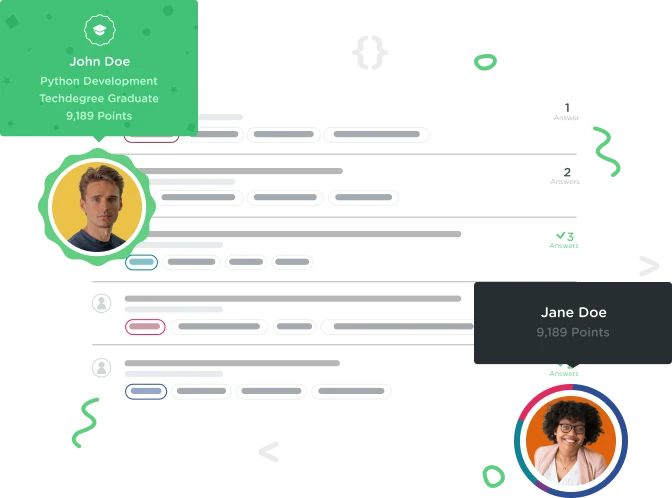

steve kevin arias serrano
9,037 Pointsi donΒ΄t know what is wrong with my code
import java.io.Console;
public class Prompter
{
private Game mGame;
public Prompter(Game game){
mGame = game;
}
public void play()
{
while(mGame.getRemainingTries()> 0)
{
displayProgress();
promptForGuess();
}
public boolean promptForGuess()
{
Console console = System.console();
boolean isHit = false;
boolean isValidGuess = false;
while (! isValidGuess) {
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
try {
isHit = mGameApplyGuess(guess);
isValidGuess = true;
} catch (IllegalArgumentException iae) {
console.printf("%s. Please try again.\n", iae.getMessage());
}
}
return isHit ;
}
public void displayProgress()
{
System.out.printf("You have a %d tries left to solve: %s\n",
mGame.getRemainingTries(),mGame.getCurrentProgress());
}
}
[MOD: edited codeblock]
1 Answer

Leandro Andrade
853 PointsYou have to create mGameApplyGuess() method
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public void play() {
while (mGame.getRemainingTries() > 0) {
displayProgress();
promptForGuess();
}
}
public boolean promptForGuess() {
Console console = System.console();
boolean isHit = false;
boolean isValidGuess = false;
while (!isValidGuess) {
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
try {
isHit = mGameApplyGuess(guess);
isValidGuess = true;
} catch (IllegalArgumentException iae) {
console.printf("%s. Please try again.\n", iae.getMessage());
}
}
return isHit;
}
//here is
private boolean mGameApplyGuess(char guess) {
return false;
}
public void displayProgress() {
System.out.printf("You have a %d tries left to solve: %s\n", mGame.getRemainingTries(), mGame.getCurrentProgress());
}
}
[MOD: edited code block]