Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial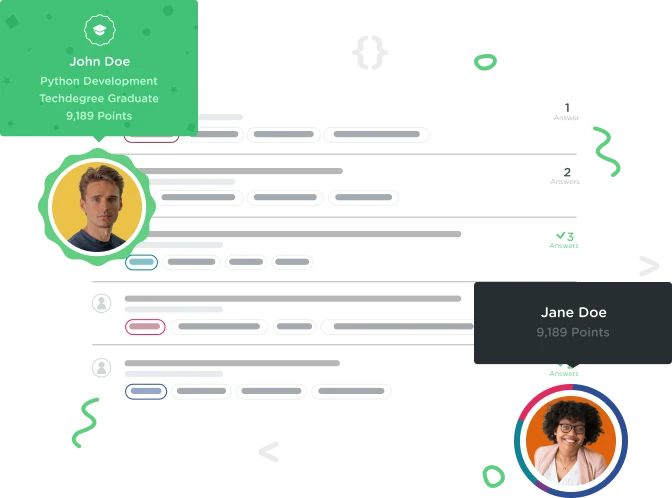

Darcy Phillips
4,087 PointsI don't fully understand the objective
Error Handling in Swift, course item no. 8. Here is the challenge:
"In the editor, you have a struct named Parser whose job is to deconstruct information from the web. The incoming data can be nil, keys may not exist, and values might be nil as well. In this task, complete the body of the parse function. If the data is nil, throw the EmptyDictionary error. If the key "someKey" doesn't exist throw the InvalidKey error. Hint: To get a list of keys in a dictionary you can use the keys property which returns an array. Use the contains method on it to check if the value exists in the array"
I don't quite understand what is meant by the "data" being nil. Does it mean that it would have a valid key, but the value would be nil (That wouldn't seem like an "empty dictionary" then)? Just not sure what I am to be checking for.
enum ParserError: ErrorType {
case EmptyDictionary
case InvalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
3 Answers
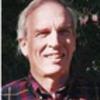
jcorum
71,830 PointsDarcy, here you go:
func parse() throws {
if data == nil {
throw ParserError.EmptyDictionary
}
if data!["someKey"] == nil {
throw ParserError.InvalidKey
}
}
If data is nil then throw the EmptyDictionary error. If there's no value for "someKey" then throw the InvalidKey error.

John Hendrix
21,844 PointsThe above has an error with the capitols. This worked for me to pass challenge 1 of 3.
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
if data == nil {
throw ParserError.emptyDictionary
}
if data!["someKey"] == nil {
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
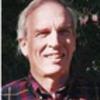
jcorum
71,830 PointsDominik, forced unwrapping is generally to be avoided, as the various TH instructors emphasize.
But note that here the first if checks to see if data is nil. So there's absolutely no way for the forced unwrapping to fail in this case.
Or, for a different example, would you say the forced unwrapping in IBOutlets is really not that good?
@IBOutlet weak var display: UILabel!
Dominik Metzger
4,959 PointsDominik Metzger
4,959 Pointsbut is a force-unwrap really that good?
Darcy Phillips
4,087 PointsDarcy Phillips
4,087 PointsOh, do the instructions mean "if the variable "data" is nil"? My confusion comes from the expression "the data",