Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial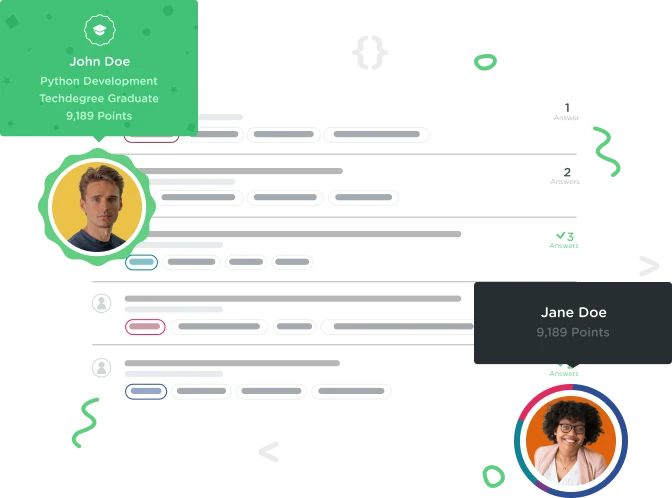

Todd Stacy
5,816 Pointsi dont get init methods
idk what im missing here. i even tried figuring this out in the playground on xcode. do i need to call an init method here? when i tried that it said i couldnt change them because the names are constants, but the way i have it now the compiler gives me "cannot invoke 'getFullName' with an argument list of type '(lastName: String)'"
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override func getFullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor.getFullName(Person.lastName)
1 Answer
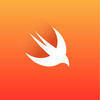
Steven Deutsch
21,046 PointsHey Todd Stacy,
The getFullName() method you created is what is known as an instance method. This means its a method that you call on an instance of the type which this method is specified in. This means, to use this method, you need to have an instance of Doctor first.
All you have to do is create an instance of Doctor using the initializer we inherited from the parent class Person and assign it to a constant named someDoctor. This challenge a little misleading because you don't actually call the instance method you created.
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override func getFullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Todd", lastName: "Stacy")
Good Luck
PS. To call this method we would call it on an instance of Doctor
someDoctor.getFullName() // will print Dr. Stacy

Todd Stacy
5,816 Pointsthank you so much Steven!
Todd Stacy
5,816 PointsTodd Stacy
5,816 Pointsi swear im trying my best.