Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial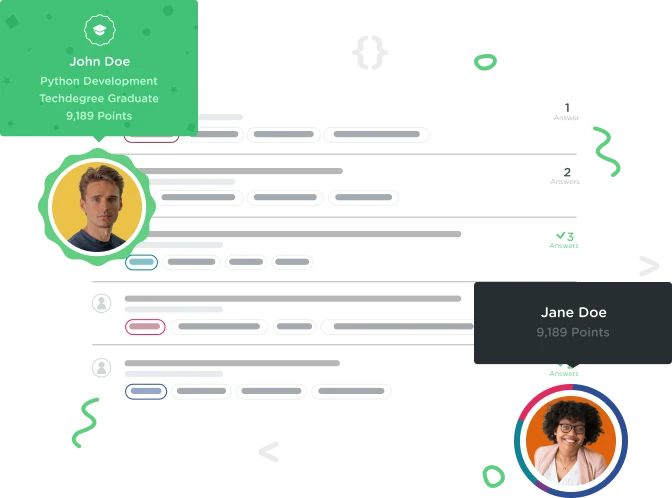
2 Answers
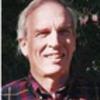
jcorum
71,830 PointsThe challenge wants you to write a second drive() method that overloads the first one, but which takes no parameters (i.e., has a different method signature) and reduces mBarsCount by 1.
public void drive() {
drive(1);
}
Rather than return mBarsCount -= 1;
, you can call the 1st method and pass it a parameter of 1, letting it do the job of decrementing mBarsCount
. This is a good, though minor, example of DRY programming, not repeating any code. This way, if you decide to change the drive() method, you only need to make the change in one place.
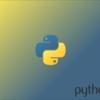
Kevin Faust
15,353 Pointshi. this is what we have so far:
private int mBarsCount;
//code omitted
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
so we have a integer variable called mBarsCount and we have a method called drive that subtracts a number from mBarsCount depending on the number passed in
what the challenge wants you to do is create another method called drive but this time, it accepts no arguments. what we have to do inside is subtract "1" from mBarsCount
take a look below
public void drive() {
mBarsCount -= 1
}
public void drive() {
drive(1);
}
You are probably more comfortable with the first method i wrote above. we simply subtract 1 from the mBarsCount. but the challenge wants you to call the pre-existing drive method which takes in arguments, and pass in 1 from there. the second way is an example of method overloading where we have more than one of the same methods but it takes different arguments. in the second one, we are also calling a method within a method.
hope that helped