Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial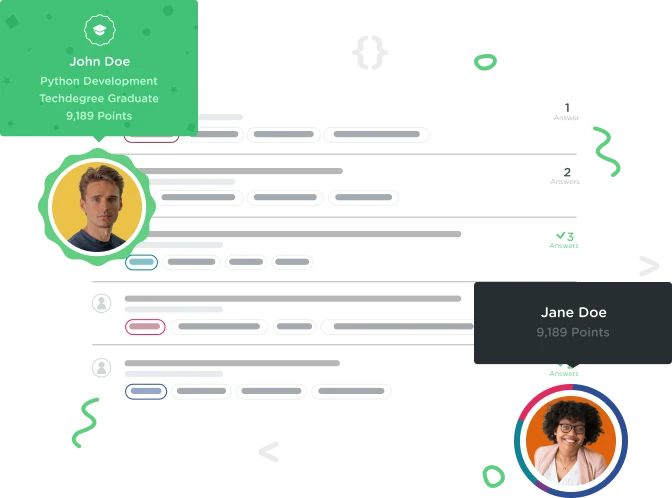

Joseph Burgan
1,312 PointsI don't get it
Can someone please explain the solution to me?
Below is the code for the last code challenge. This would work better as a do...while loop. Re-write the code using a do...while loop.
var secret = prompt("What is the secret password?");
while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
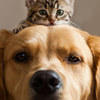
Devin Scheu
66,191 PointsFirst it asks you to use a do-while loop, and from the video before we learn the syntax looks like this:
var secret;
do {
code here
}
while ( conditional)
We know the conditional because it is the same conditional that is inside the parenthesis in the while loop.
do {
code here
}
while ( secret !== "sesame" )
Next we must tell the loop what to do inside the block of the do part. In this case we want to pop up a prompt, ask for a input and set a variable called secret. We want to put this in the body of the do part of the loop like this:
do {
secret = prompt("What is the secret password?");
}
while ( secret !== "sesame" )
Finally you need to remove the while loop from your code, also you don't need to call prompt when you set the secret variable because it is done in the loop. the final code should look like this:
var secret;
do {
secret = prompt("What is the secret password?");
}
while ( secret !== "sesame" )
document.write("You know the secret password. Welcome.");
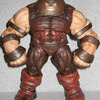
Jacie Fortune
8,969 PointsThe reason why a do-while loop is a better solution is because you actually want to ask the user for the password at least once. In the while-do, secret is undefined the first time the condition is checked. For the do-while, secret has been assign a value before the condition is checked.
alonzo alvarado
3,387 Pointsalonzo alvarado
3,387 Pointsthis code break-down save my computer from getting punched through xD thank you so much :D its still kinda hazy but i understand it more than before :D
Devin Scheu
66,191 PointsDevin Scheu
66,191 PointsYes do-while loops can be confusing at first but they prevent you from rewriting the same code over and over, saving you time and confusion later on. Remember the DRY principle! :)