Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial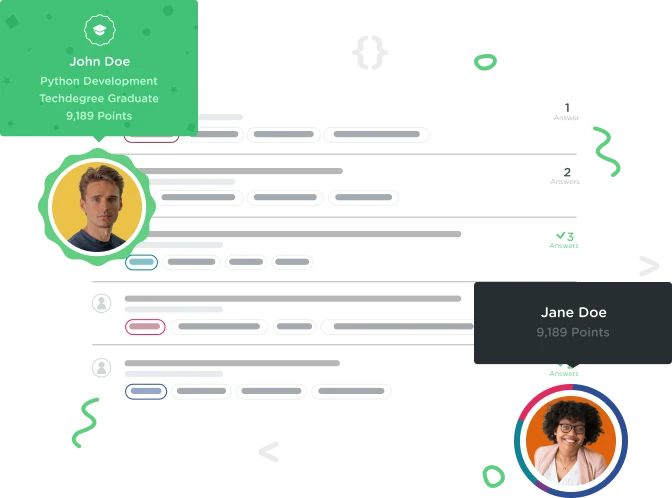

Shane Zhu
548 PointsI dont get it! I dont know whats wrong with my code=.=
plz help, what an enhanced loop
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public int getCountOfLetter(char letter){
int count = 0;
if(hasTile(letter)){
for(int i = 0; i < tiles.length(); i++){
if (tiles.indexOf(i) == letter)
count++;
}
}
return count;
}
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
3 Answers

andren
28,558 PointsThe challenge requires that you use an enchanted for loop. The way it works is that it takes the contents of an Array/List and automatically assigns each item to a variable within the loop. That means you don't have to manually pull out items using an index variable.
Here is an example:
public int getCountOfLetter(char letter){
int count = 0;
if(hasTile(letter)){
for(char tile : tiles.toCharArray()){
if (tile == letter)
count++;
}
}
return count;
}
During the first part of the for
loop declaration you specify the type and name of the variable that should hold the individual items, and then in the second part (after the colon) you specify an array or list to pull items from. The toCharArray
method is used to convert the tiles
String
to a char
array.
Edit: I originally stated that your code was technically fine, but as Balazs Pukli states that is actually not correct since the indexOf
method does not return an item by index. I missed that while glancing at your code. I have therefore removed that part of my post.
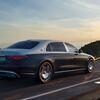
Balazs Peak
46,160 PointsFirst, the indexOf was not appropriate because that returns the index of an element, not the element by index. You were trying to use charAt there.... BUT... :)
The challenge specifies that you need to use an enhanced for loop. This is actually a foreach loop, but Java calls it enhanced for loop. You also need to use the toCharArray, because Java doesn't have iterable strings. This toCharArray takes a string, a spits an array of chars for you, which you can iterate :) Hope this helps. Much love
public int getCountOfLetter(char letter){
int count = 0;
if(hasTile(letter)
{
for (char c : tiles.toCharArray())
{if (c == letter){count++;}}
}
return count;
}

Shane Zhu
548 PointsThanks I get it! (btw the charAt seems to be useless)
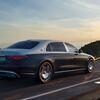
Balazs Peak
46,160 PointsIt is useless in this case - because the enhanced for loop will already extract the char out for you :)
Shane Zhu
548 PointsShane Zhu
548 PointsThanks Andren! I made it. You and Balazs Pukli have been so helpful. Thx