Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial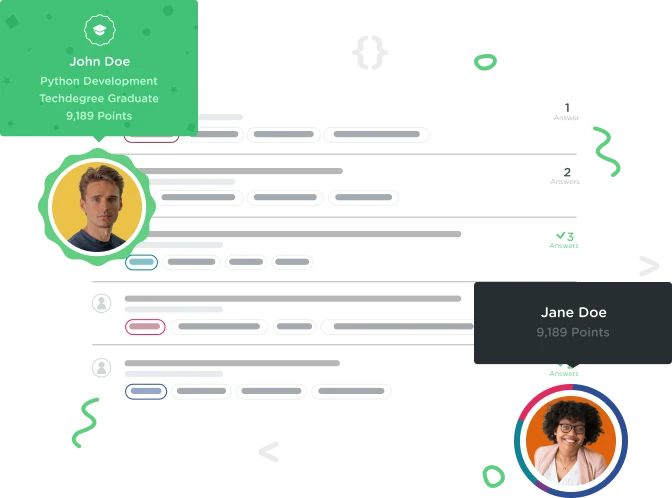

Minseok Kim
2,708 PointsI dont get it... what does raising mean?
"in order to cause an exception to happen, you use the keyword raise"...????????????
Aren't exceptions the same thing as errors? How would you cause an error to happen by using raise, if it's the inputter that makes errors happen (dividing by 0, etc.)??
3 Answers

andren
28,558 PointsAn exception is a way to communicate that an error has occurred, it is often used interchangeably with the word error because exceptions represents errors, but technically speaking they are not the same thing. Exceptions are a construct that allow you to indicate that an error has occurred along with the type of the error and potentially a message providing additional information.
While it is true that some errors are so fundamental that Python will raise an exception automatically (like with division by zero) most errors that occur in programming are of a type that Python itself cannot identity. Meaning that it is up to the functions to identity and raise the error manually.
Let's say you were writing a function that for some reason required the argument to be a number between 0
and 100
(inclusive). Any higher or lower than this would not work with what the function was intended to do. How would you ensure that the value passed in met those criteria? Python itself would have no issue with your function being called with a value like 101
since that is an entirely valid value to pass into a function.
The answer is that you would setup a conditional statement that checked if the value was within the expected range, if it was not you would raise an exception (likely a ValueError
in this case) to indicate that a problem had occurred. This exception would then be thrown up to the function that called the function where the exception occurred where it can be dealt with through a try
statement.
Here is an example of how such a function would look:
def exception_example(number):
if number < 0 or number > 100:
raise ValueError("Passed in number needs to be between 0 and 100 (inclusive)")
# Then imagine lots of code under here that actually did something with the number
In that hypothetical case the error would not actually occur within the function. It would occur when the function was called with an invalid number, but there is no way for the function itself to prevent that from happening. And while you can setup checks for this before you call the function, this function could be one that is called many places in your program and gets input from multiple sources (user input, reading from a file, retrieved from a server, etc) so having input validation inside of the function itself makes more sense than to rely on the assumption that the function will never be called with an incorrect value at any point.
Once an exception has been raised it can be dealt with by other code in your program, in this case the program could ask for the number again. You are also not limited to the built-in exceptions, you can create custom exception types if your code has some error cases that Python's built-in exceptions has not accounted for.
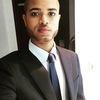
Jackie Santana
7,403 Pointsandren you're just giving another example if what craig dennis did, you're still not explaining what is the the point of raise if you already had expections?, so for all that why do we have to put expections if we already have a raise? vice versa, im confused .

andren
28,558 PointsI don't quite understand your question. Raise is just a keyword used to trigger an exception. It wouldn't have any purpose if it was not paired with an exception, and conversely exceptions would have no purpose if there was no way to trigger them from your code, to indicate that the error described by the exception has occurred.
When you get an exception from a built-in function like int()
that was also triggered with a raise
within the function.
So raise
and exceptions depend on eachother. One way of looking at it is that exceptions defines error states, and raise allows you to indicate that said error state has occurred.

Peter Jacobsen
2,142 PointsI see raise as, when you raise your hand in school you want teacher ot pay attention to you so you can ask a question or give an answer. Or if you raise a banner fighting for rights etc. you want attention. And we don't want people to try and bug our program so they must input a higher number than 1 since 1 person can't split the check by himself. So we raise a ValueError so program knows "okay, they (developer) want me to pay attention to the input and make sure its correct, if its not correct i will tell the useres theres a ValueError and give them the developers message for that error"