Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial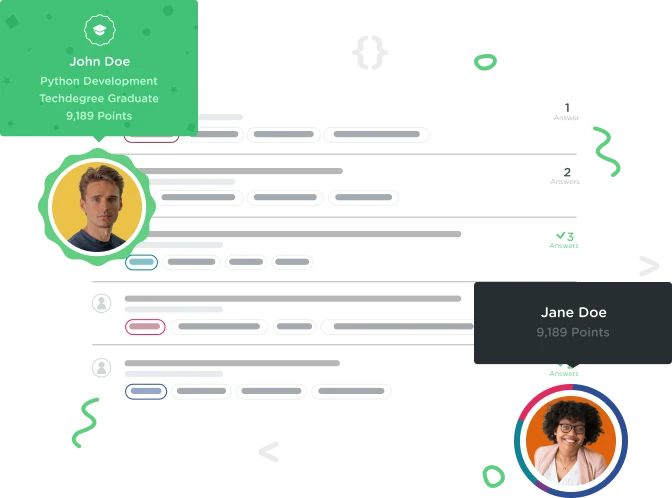

Roshan Kashif
2,976 PointsI don't get this
what do I do
package com.example.model;
import java.util.Map;
import java.util.Set;
import java.util.HashMap;
public class Contact {
private String mFirstName;
private String mLastName;
private Map<String, String> mContactMethods;
public Contact(String firstName, String lastName) {
mFirstName = firstName;
mLastName = lastName;
/* This stores contact methods by name
* eg: "phone" => "(555) 555-1234"
*/
mContactMethods = new HashMap<String, String>();
}
public void addContactMethod(String method, String value) {
// TODO: Add to the contact method map
}
/**
* Returns the available contact methods. eg: phone, pager,
*
* @return The name of the contact methods that are available
*/
public Set<String> getAvailableContactMethods() {
// FIXME: This should return the current contact method names.
return null;
}
/**
* Returns the value for the contact method if it exists,
*
* @param methodName The name of the contact method to look up.
* @return The name of the contact methods that are available
*/
public String getContactInfo(String methodName) {
// FIXME: return the value for the passed in *methodName*
return null;
}
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
}
1 Answer
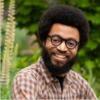
Nicolas Hampton
44,638 PointsAlright, we're creating and object called "contacts". Who's contacts are they and what can we use to contact them. Well, each contact is made with the constructor, the necessary data (or state) to create this object. Well, each contact in my phone has a name, a method of contact, and the specific kind of contact it is. In this constructor, we have variables for the first and last name, and a map. Why would we need a map? Well, in my phone, I can contact John Doe via email, phone, or facebook. John's email is nobody@noone.com, his phone is 222-222-2222, and his facebook handle is funnilookin. Well, a map attaches a key (such as the type of contact, phone) with a value (a phone number, or 222-222-2222). Think about this constructor until you get a graps of the object we're manipulating and what comes next will come a little easier.
The first thing we have to do is finish the addContactMethod method. A contact method is aphone and a number. Just like the blank map in the constructor. So we're PUTing a key and value into the contact. How do we do that. Go to the documentation to find out: https://docs.oracle.com/javase/7/docs/api/java/util/Map.html Remember, if we're doing something right, it shouldn't take much code at all.
The next method asks us to get all the contacts methods for a particular contact in our phone, or getContactMethods. Well, since the guy made that comment in the constructor that says that that he want's to put each contact method into the key, we just need a list (or maybe a Set, since that's what the method is returning) of all the keys in the map. A lot of this is working and thinking about abstract, imaginary things, and thinking about them like they're real in the most simple of terms. If you don't know how to get a list of keys, just ask the documentation. That gets to be the easy part. The abstraction is what a coder is made of (I think).
The last method is getContactInfo. We enter in phone, and return 222-222-2222. With all the rest of the problems out of the way, you should be able to handle this. Just model it with the rest you just did, look up how to do it, and keep it simple.
Good luck, feels great when you get it, believe me. Nicolas
Roshan Kashif
2,976 PointsRoshan Kashif
2,976 PointsThanks Nicolas, I got through the the first 2 but the third part of the challenge is kind of hard aren't you just meant to return the method name.
Nicolas Hampton
44,638 PointsNicolas Hampton
44,638 PointsRoshen,
It is pretty simple, but your skipping a step. the argument we're sending into the method is methodName. This is meant to be phone, or email, or work phone or whatever. We made a map for these, with the methodName being the Key, and the value being, well, the value. In the documentation for the Map interface, there's a method named get(), that will get the value attached to a key in the Map. So, in order to get the number for a phone, the code would look like this:
return mContactMethods.get(methodName);
Just that simple. Just remember not to be so concerned with the code, or memorization, so much as mastering the basic syntax it's ordered in, and how to translate an idea, or object, into code syntax. If you understand what's being said and created, you might still come across a roadblock, but it will be the right roadblock, and easily solved with a little documentation lookup.
:) Nicolas
Roshan Kashif
2,976 PointsRoshan Kashif
2,976 PointsThank a lot now I get the whole challenge