Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial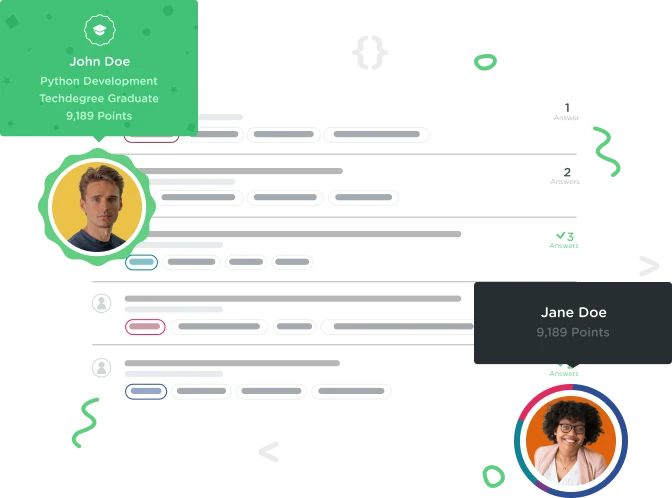

ISAIAH S
1,409 PointsI don't get this challenge
So I was wondering if you could help me with some of these code challenges. As you might've seen when a member field name doesn't match the style we've talked about, I send an error about it. Let's write some code to validate the style of the field name.
I've added the method validatedFieldName it will return the validated field name. If the value passed in doesn't meet the requirements, throw an IllegalArgumentException. I'll keep you posted on the results every time you press check work.
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
return fieldName;
}
}
9 Answers
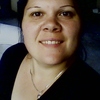
Annamaria Toth
6,617 PointsIn you second if statement you have to define that the second character must be an upper case and must be a letter.
if ( Character.isLowerCase(secondChar) || Character.isLetter(secondChar)
It did pass for me.

ISAIAH S
1,409 PointsYou mean
if ( Character.isLowerCase(secondChar) || !Character.isLetter(secondChar)

Geoff Parsons
11,679 PointsYou need to check if the fieldName
string passed in to the function matches the requirements. If it does return fieldName
unaltered, if it does not you need to throw an IllegalArgumentException.

ISAIAH S
1,409 PointsHow?
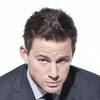
Ivan Knyazev
4,927 PointsIn first case you must check if the fieldName first character is equal to 'm' character if it's not then throw an exception like Craig did in previous lesson. In Second case first of all you must create a char variable to store second character of fieldName(remember counting begins from 0) for example secondChar then you should check if your new char variable secondChar(that is already stores second character of fieldName) has upperCase character..if it's not then throw an exception

ISAIAH S
1,409 PointsI tried:
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
char secondChar = fieldName.charAt(1);
if (fieldName.charAt(0) != 'm' || secondChar.isLowerCase)
{throw new IllegalArgumentException( fieldName + " is invalid.");}
return fieldName;
}
}
. . .But it said the char can't be dereferenced.
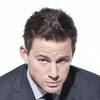
Ivan Knyazev
4,927 PointsThis is how you should do: Character.isLowerCase(secondChar)
You can read more about this features in java documentation as Craig in previous lessons.

ISAIAH S
1,409 PointsNow it's saying "Bummer! Expected "m_first_name" to fail but it passed."
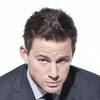
Ivan Knyazev
4,927 PointsDid you passed this chalange?

ISAIAH S
1,409 PointsNo.
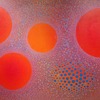
Jef Davis
29,162 PointsI checked your code and ran into the same problem you're reporting when I tested it out. When I passed this challenge, I separated out the IllegalArgumentException into two separate if statements. I like the economy of your code, but I think trying to be so concise has lead you to the problem you're currently facing.
We have two objectives: throw an IllegalArgumentException if 1) The first character of the string does not equal 'm' and if 2) the second character of the string is not a letter OR is lower case
If you write these two objectives as separate if statements, you might have better results
http://docs.oracle.com/javase/7/docs/api/java/lang/Character.html

ISAIAH S
1,409 PointsI tried:
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
char secondChar = fieldName.charAt(1);
if (fieldName.charAt(0) != 'm')
{throw new IllegalArgumentException( fieldName + " is invalid.");}
if (Character.isLowerCase(secondChar)) {throw new IllegalArgumentException( fieldName + " is invalid.");}
return fieldName;
}
}
But I am still getting the same thing ( Bummer! Expected "m_first_name" to fail but it passed. ).
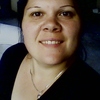
Annamaria Toth
6,617 PointsYes, sorry that`s what I meant.

ISAIAH S
1,409 PointsThanks!!
It worked!!

Rock Hudson
27,595 PointsThe issue wasn't the fact that you needed to separate it into two if statements, the issue was the logic... and that you weren't checking to see if it was NOT a letter... the following code passes:
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
char secondChar = fieldName.charAt(1);
if (fieldName.charAt(0) != 'm' || Character.isLowerCase(secondChar)
|| !Character.isLetter(secondChar)) {
throw new IllegalArgumentException();
}
return fieldName;
}
}
Ethan Lowry
Courses Plus Student 7,323 PointsEthan Lowry
Courses Plus Student 7,323 PointsWhat don't you get, specifically? What have you tried so far? The challenge wants you to take the 'fieldName' variable passed into this method, and then perform the actions described in the comments on it, before returning it.