Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial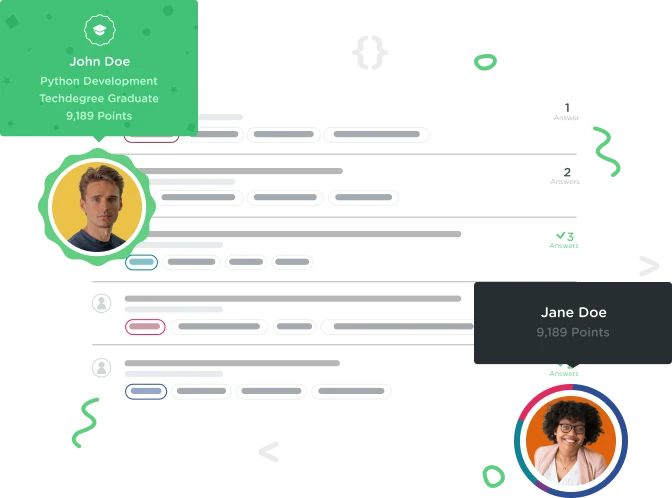

Einars Vilnis
8,050 PointsI don't get what i'm do wrong in phyton objects score challenge
I think that my code is good and I did test it and it worked. so where is the problem and why system is not accepting the answer?? thank you guys allready
class Game:
def __init__(self):
self.current_score = self.score()
def score(self):
playeScore = [0, 0]
player = input("1, or 2: ")
if player in '12':
if player == '1':
playeScore[0] += 1
return playeScore
else:
playeScore[1] += 1
return playeScore
else:
return player
6 Answers
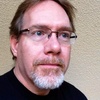
Chris Freeman
Treehouse Moderator 68,454 PointsI think you were reading more complexity into the challenge. The object oriented python course score method challenge asks:
Add a score
method to Game
that takes a player argument that'll be either 1 or 2. Increase that player's value in self.current_score
by 1. You'll need to adjust the index (i.e. player = 1
means self.current_score[0]
needs to increase).
Breaking that down. "Add a score
method to Game
...." You got that correct by adding:
def score(self):
"...that takes a player argument...." Here you needed to add an argument to the method:
def score(self, player):
"... that'll be either 1 or 2. Increase that player's value in self.current_score
by 1. You'll need to adjust the index (i.e. player = 1
means self.current_score[0]
needs to increase). "
See comments in the code:
class Game:
def __init__(self):
self.current_score = [0, 0]
def score(self, player):
# Check if player value is 1
if player == 1:
# using index 0, increase player 1 score by 1
self.current_score[0] += 1
# Check if player value is 2
elif player == 2:
# using index 1, increase player 2 score by 1
self.current_score[1] += 1
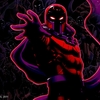
Iwan Morgan
11,257 PointsAnother way to do it is:
def self(self, player):
player -= 1
self.current_score[player] += 1
Not really protecting yourself from arguments that isn't 1 or 2 though.

Shaohui Yang
5,009 Pointsdef score(self, player): if player == 1 or player == 2: self.current_score[player - 1] += 1
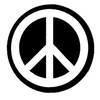
john larson
16,594 PointsI like how you did that

Thorbjørn Bak Jensen
15,043 Pointsin one line: def score(self, player): self.current_score[player - 1] += 1
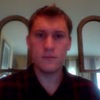
Casey Huckel
Courses Plus Student 4,257 PointsI'll try that again:
class Game:
def __init__(self):
self.current_score = [0, 0]
def score(self, player):
if player == 1:
self.current_score[0] += 1
elif player == 2:
self.current_score[1] += 1
[MOD: added ```python formatting -cf]
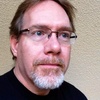
Chris Freeman
Treehouse Moderator 68,454 PointsCheck your indentation.
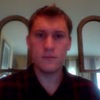
Casey Huckel
Courses Plus Student 4,257 PointsNevermind I got it by entering this:
class Game:
def init(self): self.current_score = [0, 0] self.player = 1
def score(self,player = 1): self.player = player if self.player == 1: self.current_score[0] += 1 elif self.player == 2: self.current_score[1] += 1 return self.current_score
Casey Huckel
Courses Plus Student 4,257 PointsCasey Huckel
Courses Plus Student 4,257 PointsI tried this but got "Did you create the 'score' method?
class Game: def init(self): self.current_score = [0, 0]