Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial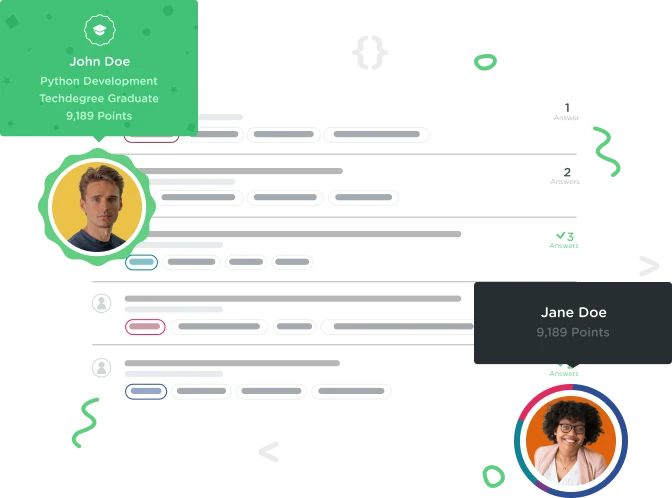

Josephine Gonzalez
1,868 PointsI don't get what's wrong??
public class Game {
public static final int MAX_MISSES = 7;
private String mAnswer;
private String mHits;
private String mMisses;
private String letter;
public Game(String answer) {
mAnswer = answer;
String mHits = "";
String mMisses = "";
}
private char validate(char letter){
if (! Character.isLetter(letter)){
throw new IllegalArgumentException("a letter is required");
}
letter = Character.toLowerCase(letter);
if (mMisses.indexOf(letter) >=0 || mHits.indexOf(letter) >=0) {
throw new IllegalArgumentException(letter + " has already been guessed");
}
return letter;
}
public boolean applyGuess(char letter) {
letter = validateGuess(letter);
boolean isHit = mAnswer.indexOf(letter) >= 0;
if (isHit) {
mHits += letter;
} else {
mMisses += letter;
}
return isHit;
}
public String getCurrentProgress() {
String progress = "";
for (char letter : mAnswer.toCharArray()) {
char display = '-';
if (mHits.indexOf(letter) >= 0) {
display = letter;
}
progress += display;
}
return progress;
}
public int getRemainingTries() {
return MAX_MISSES - mMisses.length();
}
}
./Game.java:27: error: incompatible types: boolean cannot be con
verted to char
letter = validateGuess(letter);
^
1 error
2 Answers

Craig Dennis
Treehouse TeacherI think you want to call validate
and not validateGuess
(which I actually don't see here).
It seems that validateGuess
is returning a boolean, but you are attempting to store that in letter, which is a char
. That is what the type conversion error is talking about.
(I wrapped your code in 3 backticks followed by java so I could get the pretty color coding)
Let me know if that fixes it, I think it will!

Josephine Gonzalez
1,868 PointsIt solved the problem. Thanks!