Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial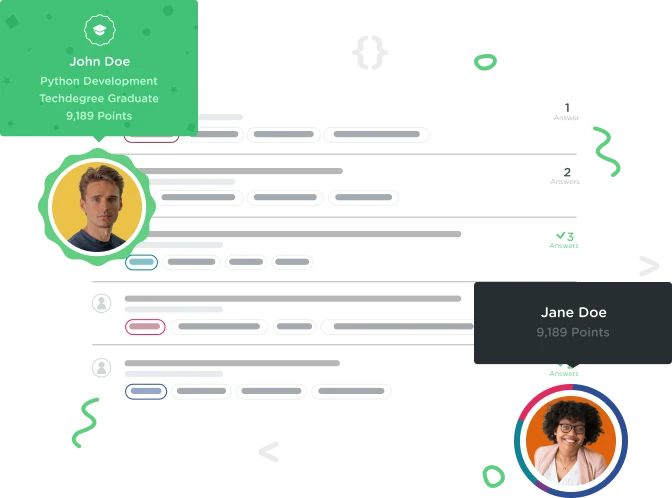

Calvino Miguel
4,337 PointsI dont' get why I'm not getting the default message of my Switch Statement
I had a role variable that was defined like this:
$role = 'subscriber';
then I created a switch statement.
switch ($role != 'admin') {
case 'admin': echo 'As an admin, you can add, edit, or delete any post.'; break;
default:
echo 'You do not have access to this page. Please contact your administrator.';
break;
}
I expected the "You do not have access to this page. Please contact your administrator." to be echoed, but the other case was being echoed the whole time. And when I assign the string 'admin' to the variable $role, I get the default message.
I thought that a switch statement works the same as a if statement. In this case the if statement would be something like:
if ($role != 'admin'){ echo 'You do not have access to this page. Please contact your administrator.'; } else { echo 'As an admin, you can add, edit, or delete any post.'; }
<?php
//Available roles: admin, editor, author, subscriber
$role;
if (!isset($role)) {
$role = 'subscriber';
}
//change to switch statement
switch ($role != 'admin') {
case 'admin':
echo 'As an admin, you can add, edit, or delete any post.';
break;
default:
echo "You do not have access to this page. Please contact your administrator.";
break;
}
3 Answers
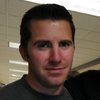
Adam Pengh
29,881 PointsFor a switch statement, you only put the variable to be evaluated. Then each case is equivalent to an "if" statement.
<?php
if ($i == 0) {
echo "i equals 0";
} elseif ($i == 1) {
echo "i equals 1";
} elseif ($i == 2) {
echo "i equals 2";
}
switch ($i) {
case 0:
echo "i equals 0";
break;
case 1:
echo "i equals 1";
break;
case 2:
echo "i equals 2";
break;
}
?>

Calvino Miguel
4,337 PointsThank you Adam, now it makes sense to me :)
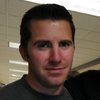
Adam Pengh
29,881 Points<?php
//Available roles: admin, editor, author, subscriber
if (!isset($role)) {
$role = 'subscriber';
}
//change to switch statement
switch ($role) {
case 'admin':
echo 'As an admin, you can add, edit, or delete any post.';
break;
case 'editor':
echo 'As an editor, you can add or edit any post, and delete your own posts.';
break;
case 'author':
echo 'As an author, you can add, edit, or delete your own post.';
break;
default:
echo "You do not have access to this page. Please contact your administrator.";
break;
}

Calvino Miguel
4,337 PointsHi Adam, thank you for taking your time to help me. I have already cleared the exercise. And I wrote exactly the same thing that you did and it worked out. The thing is that I have the impression that I didn't really understand how the switch statement works. Can you explain to me what happens when I write switch ($role != admin) ? What does the server process when I write that ? Thank you for your help!