Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial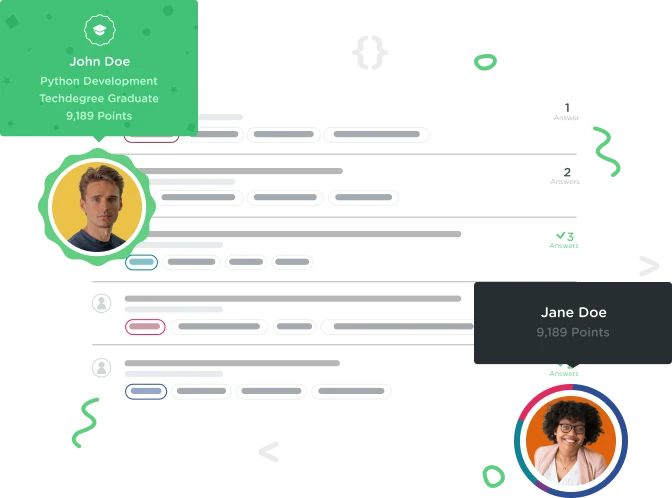

Andrei Oprescu
9,547 PointsI don't get why the code skips letters?
So, while doing a task on a course, I stumbled across this question:
OK, I need you to finish writing a function for me. The function disemvowel takes a single word as a parameter and then returns that word at the end.
I need you to make it so, inside of the function, all of the vowels ("a", "e", "i", "o", and "u") are removed from the word. Solve this however you want, it's totally up to you!
Oh, be sure to look for both uppercase and lowercase vowels!
And the code that I am using is at the bottom of the comment.
The problem is that when I submit the code, it gives me an error saying:
e.g.
Bummer! I called disemvowel('AaTOOL') and I expected to get in return 'TL', but I got 'aTOL'
Can someone tell me what I did wrong?
Thanks
vowels = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
def disemvowel(word):
word_letters = list(word)
for letter in word_letters:
if letter in vowels:
word_letters.remove(letter)
return ''.join(word_letters)
3 Answers
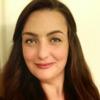
Jennifer Nordell
Treehouse TeacherHi there! The problem here is that you're mutating the thing that you're iterating over. Essentially, it's messing up the indexing of the list. The remove removes the item you're looking at the following letter falls down into its place without being checked. The end result is that whenever you use remove
to remove something from that list, the next item will not be checked. This isn't a problem when the next item is a consonant, but when it's a vowel, it becomes a problem. To mitigate this, you can make a copy of the list and then either iterate over the original and mutate the copy, or iterate over the copy and mutate the original. It will need to be the mutated version that is then joined and returned.
Hope this helps!

Andrei Oprescu
9,547 PointsOh thanks! I understand what the problem was now and I solved it.
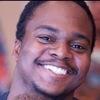
Clinton Johnson
28,714 PointsAndrei Oprescu Can you share how you solved the problem

Andrei Oprescu
9,547 PointsI used this code:
vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
def disemvowel(word):
word_letters = list(word)
letters = []
for letter in word_letters:
if letter in vowels:
letters.append(letter)
for letter in letters:
word_letters.remove(letter)
word2 = ''.join(word_letters)
return word2