Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial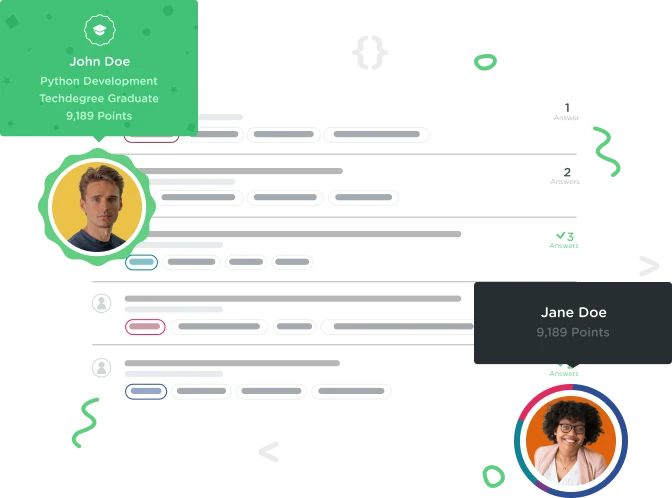
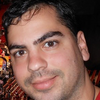
Angel Naranjo
30 PointsI don't get why you put attempt_count += 1 inside the if block. Isn't this equal to 2?
import sys
password = input("Please enter the super secret password: ") attempt_count = 1 while password != "opensesame": if attempt_count > 3: sys.exit("Too many invalid password attempts") password = input("Invalid password, try again: ") attempt_count += 1
print("Welcome to secret town")
2 Answers
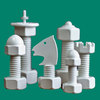
Steven Parker
230,274 PointsThe statement "attempt_count += 1
" will increase the value in "attempt_count" by one. So if it begins as 1, the first time this statement is encountered, it will change to 2. But the next time it will become 3, and then 4, as the loop continues to repeat.
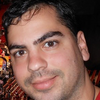
Angel Naranjo
30 PointsWhy is it that I get 4 attempts when I write the code this way:
import sys
password = input("Please enter the super secret password: ")
attempt_count = 1
while password != "opensesame":
if attempt_count > 3:
sys.exit("too many invalid password attempts")
password = input("Invalid password, try again: ")
attempt_count += 1
print("Welcome to secret town")
It will let you get up to 4 wrong answers, but if I write like this:
import sys
password = input("Please enter the super secret password: ")
attempt_count = 1
while password != "opensesame":
attempt_count += 1
if attempt_count > 3:
sys.exit("too many invalid password attempts")
password = input("Invalid password, try again: ")
It gives me 3 attempts.
I reversed the attempt_count += 1
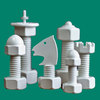
Steven Parker
230,274 PointsThe difference is the first example tests to see if the value is more than 3 before it changes it; But in the second example it changes it first, and then tests to see if it is more than 3.
So because of the order, the first example repeats one more time than the second one.
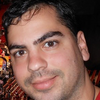
Angel Naranjo
30 PointsIf this code were to go first:
if attempt_count > 3:
The value for attempt_count can instantly reach 4 attempts by the user and be greater than 3.
However, if this code were to go first:
attempt_count += 1
The value for attempt_count would be the number of attempts by the user, which would max out at 3 because adding 1 will make it 4. Then it follows with the if attempt_count > 3: code.
Thanks, Steven
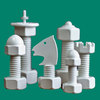
Steven Parker
230,274 PointsI'm not sure what you mean by "instantly". The loop still must repeat several times before the value is larger than 3.
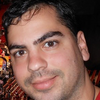
Angel Naranjo
30 PointsYes, you are correct. "Instantly" was not the proper wording. The code will repeat until the number of attempts is greater than 3.

Dayne Stevens
Courses Plus Student 5,849 Pointsattempt_count += 1 would execute after an attempt, at some point prior to this section of code you would have attempt_count = 0 most likely. So attempt_count += 1 would set it to 1 the first time it is triggered, 2 the second time and so on.
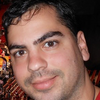
Angel Naranjo
30 PointsWhy is it that I get 4 attempts when I write the code this way:
import sys
password = input("Please enter the super secret password: ")
attempt_count = 1
while password != "opensesame":
if attempt_count > 3:
sys.exit("too many invalid password attempts")
password = input("Invalid password, try again: ")
attempt_count += 1
print("Welcome to secret town")
It will let you get up to 4 wrong answers, but if I write like this:
import sys
password = input("Please enter the super secret password: ")
attempt_count = 1
while password != "opensesame":
attempt_count += 1
if attempt_count > 3:
sys.exit("too many invalid password attempts")
password = input("Invalid password, try again: ")
It gives me 3 attempts.
I reversed the attempt_count += 1

fady mohamed
5,972 PointsHey Angel,
Yep it gives 4 attempts because we used
if attempt_count > 3
if you wanted to have it only allow 3 attempts, use >= 3 or you could use >2.
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsHello Angel,
It is difficult to read your code because of the formatting. Please read the Markdown Cheatsheet to learn how to show your code in forum posts. It is below the "Add an Answer" area