Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial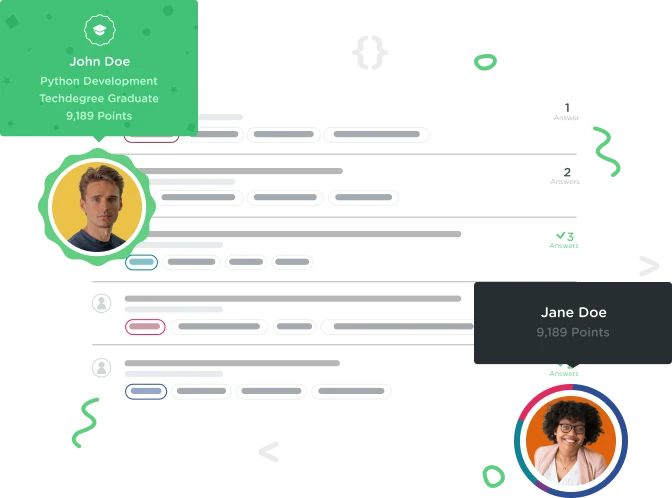

Piyut Dyoni
Android Development Techdegree Student 1,981 PointsI dont know hoe to solve this.. :(
Sorry I am newbie here... I am not sure with the question and I am pretty confused to solve this.. could you help me?
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
def word_count(string):
string=string.lower().split(" ")
i=0
dictionary={}
for item in string:
dictionary.update({item:i})
i+=1
return dictionary
2 Answers
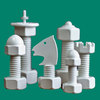
Steven Parker
231,269 PointsYou probably just need to follow the hints in the comments more closely. For example:
- In a for loop of that list, you'll have a word that you can check for inclusion in the dict (with "if word in dict"-style syntax)
You have a good for loop, but you still need to test each item to see if it's already in the dictionary. Then, they say:
- Or add it to the dict with something like word_dict[word] = 1
It's OK to substitute the update method for this suggestion, but the new item needs to start with a count of 1 instead of the current word number (i). In fact, you probably don't need to keep track of the number of words at all.
But you still need to handle the other case where the word has already been added. In that case, just raise the count.
Once you fix these issues, you should be in good shape.

Unsubscribed User
7,260 PointsI gave it a shot myself but I am completely and utterly stuck after trying for 2 hours. Here's what I believe was the closest to the solution I could get:
string = 'I am that I am'
dict = {}
def word_count():
my_string = string.lower().split()
for word in my_string:
if word not in dict:
dict.update({word: my_string.count(word)})
return dict
I do not understand why the above is not correct. When I try it in the shell myself, the dict is correctly populated.
Edit: The return was wrongly indented.. :') My god did it took a long time to find this out.. It is extremely frustrating when all you see is "Bummer, please try again" without any kind of direction. If there would be one thing that needs addressing on this site, it would be that.
Piyut Dyoni
Android Development Techdegree Student 1,981 PointsPiyut Dyoni
Android Development Techdegree Student 1,981 PointsI solved it, thank's anyway :)