Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial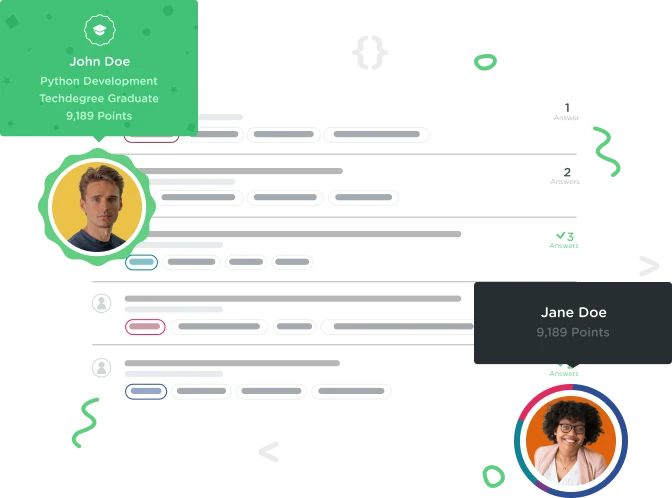

rovelle trinidad
390 PointsI don't know how to create a string
I'm tried doing this a few times already
var myName = 'your name';
var myAge = 27
var message = 'myName';
var message = 'myAge';
message = ('my name is Jake, ' + ' I am 27 '.');
1 Answer
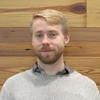
Cody Selman
7,643 PointsHello Rovelle,
When creating a variable, you only need to use the keyword "var" the first time the variable appears. In your code, you have "var" before the variable named message two times. You can remove "var" from the second line with the message variable.
It looks like you're trying to construct a string that will say "My name is Jake, I am 27 years old." We'll want to get that string to use your name and age, and also make use of the myName and myAge variables.
The first step is to change the values of the myName and myAge variables to match your real name and age. Here's an example of what you need to do to the first two lines of code, using my name and age:
var myName = 'Cody';
var myAge = 28;
Next we'll want to declare the message variable and start it off as an empty string:
var message = '';
Now we have all of the variables we need, so we can start to build the string using our variables. I always find it a bit easier to read if we break the message up into multiple lines. Here's an example of how to build up this string across multiple lines, so that adding in the myName and myAge variables will be easier:
message += 'My name is ';
message += 'Cody'
message += ', I am ';
message += 28;
message += ' years old.';
We don't want the myName and myAge variables to go to waste, so we can substitute the name and age in the code above with our variables.
message += 'My name is ';
message += myName;
message += ', I am ';
message += myAge;
message += ' years old.';
Now if we console.log(message), we should get a string that says "My name is Cody, I am 28 years old." You can replace the values of the myName and myAge variables with your name and your age, and the code will work how you've intended. I hope seeing the code written and explained this way helps make it clearer for you.

rovelle trinidad
390 PointsThank you for the very thorough explanation! I really appreciate this! Thank you again!
Juan Naputi
1,772 PointsJuan Naputi
1,772 Points1) For strings, you do not surround them with parenthesis. To combine them, you just need to use '+' to get them to combine. Here is an example (p.s. I'm going to be using the 'let' keyword for variable declarations, but you can replace it with 'var'):
For the challenge, it just requires that you create a variable
myName
and assign it your name. To get through your challenge, it is simply `var myName = 'your_name_here'. But, if you are looking to combine strings to create one string, then string concatenation using the '+' operator is all you need.If you don't want to do it that way, the alternative is to use string interpolation:
Notice how the string is not surrounded by single quotes, but by back-ticks.
Hope this helps! I can provide more explanation if needed.