Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial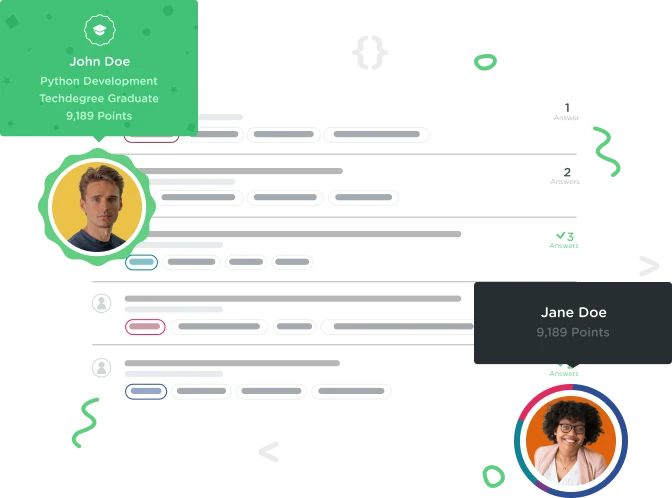

Jenna Cooper
1,195 PointsI don't know how to do this
I am supposed to add a # symbol and lastName in uppercase to the end of the userName string. The final value of userName is "23188XTR#SMITH". I am getting the wrong answer, and I don't know how to do it.
var id = "23188xtr";
var lastName = "Smith";
var userName = id.toUpperCase();
userName += "#" + lastName;
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
1 Answer
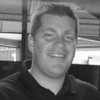
Mike Hickman
19,817 PointsHi Jenna,
You have the right idea, but you aren't making the lastName uppercase as well in this code. Probably the simplest way to do this would be to do something like:
var id = "23188xtr";
var lastName = "Smith";
var userName = id.toUpperCase() + "#" + lastName.toUpperCase();
This will give you the correct answer, because it looks like they want everything to be uppercased. Another way to do this, which is kind of what you have, would be to make a little extra code and uppercase both items before you do your final var userName
:
var id = "23188xtr";
var lastName = "Smith";
var upperID = id.toUpperCase(); // or whatever you want to call the variable
var upperName = lastName.toUpperCase();
// now that everything is uppercase, you can just add (concatenate) them together
var userName = upperID + "#" + upperName;
I know this code is a one-off, but if you were building a real project, you'd want to think about if you were going to need the uppercased ID and lastName in various spots across your code. If you'd be using it a bunch, it would be smarter to do things the second way, so you always have an uppercase ID and lastName to refer to whenever you want it, without having to run .toUpperCase() on those every single time you want to put them somewhere in your code.
Hope this helps you understand it a little bit better. Have fun!
Mike