Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial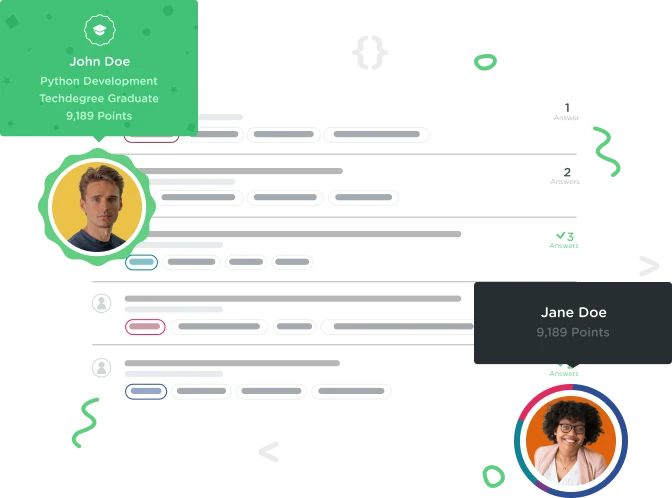

william fashanu
2,108 PointsI dont know how to tackle this question
In the editor, I’ve declared a struct named RGBColor that models a color object in the RGB space.
Your task is to write a custom initializer method for the object. Using the initializer assign values to the first four properties. Using the values assigned to those properties create a value for the description property that is a string representation of the color object.
For example, given the values 86.0 for red, 191.0 for green, 131.0 for blue and 1.0 for alpha, each of the stored properties should hold these values and the description property should look like this:
"red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0"
Note: Init methods typically list parameters in the same order of property declaration. For this task, stick to the order red,green,blue,alpha.
struct RGBColor { let red: Double let green: Double let blue: Double let alpha: Double
let description: String
// Add your code below
}
3 Answers
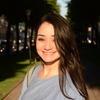
Tassia Castro
iOS Development Techdegree Student 9,170 PointsHi Willian,
Try this..
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
Hope this helps.
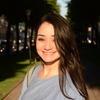
Tassia Castro
iOS Development Techdegree Student 9,170 PointsHi Willian,
The init method is simply used to assign values to the properties of the classes.
As you can see, we have a struct in this example. Structs receive a init method automatically. So this code would also work:
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
}
When you tried to create an instance of RGBColor, swift would use the automatic init and you would be able to initialise it like that.
var color = RGBColor(red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0, description: "red: 86.0, green: 191.0, blue:131.0, alpha:1.0") //automatic init
But if I am not mistaken one of the main reasons of this exercise is to make us use string interpolation. For this reason, we had to create a custom initialiser that does not receive the property description but Instead we have to create the value of the property description using the values that are being passed through the init.
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)" // this is string interpolation, when you concatenate string with a variable.
}
}
We have to agree that the second version is better. With the automatic init, every time I create an instance of RGBColor I have to pass all the values by myself to the description property. However with the custom init, the property description is always initialised for me.
var color = RGBColor(red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0) //custom init
Hope this helps.
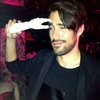
Adrian Torrente Tenreiro
12,540 PointsThe Compilator is always driving me crazy as sometimes it works the way i do it but its not set up for all possibilites
william fashanu
2,108 Pointswilliam fashanu
2,108 Pointsthank you can you explain the init method to me i dont quite understand it fully and its purpose