Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial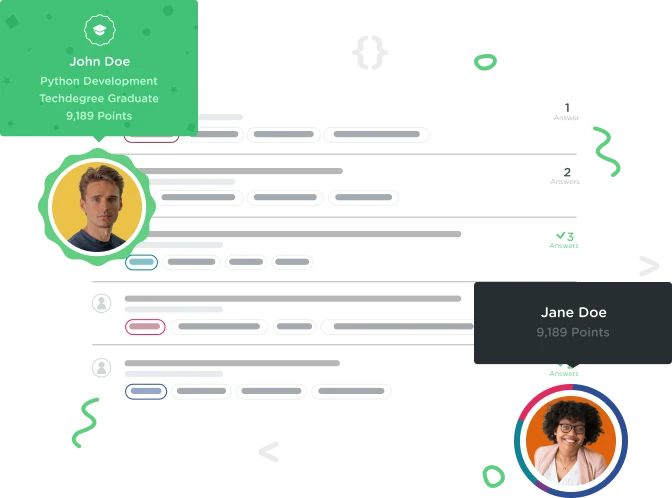

Weston Ross
832 PointsI don't know how to use the helper method correctly.
I used the coursework exercises as a guide to solving this problem and I am not understanding how to allow it to keep charging until fully charged. Thank you.
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public boolean charge(){
boolean isFullyCharged = false;
if (!isFullyCharged()) {
mBarsCount++;
isFullyCharged = true;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
}
2 Answers

Ken Alger
Treehouse TeacherWeston;
Welcome to Treehouse!
Challenge Task
Okay, so let's use our new
isFullyCharged
helper method to change our implementation details of thecharge
method. Let's make it so it will only charge until the battery reports being fully charged. Let's use the!
symbol and awhile
loop. Inside the loop incrementmBarsCount
.
The section of code we will be concentrating on is:
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
The challenge is asking us to use a while
loop, which if you recall has a general syntax of while ( condition ) { do something }
. Since we are charging a battery, we only want to do keep charging it if the battery is not fully charged. How can we check that? That is the reference to the isFullyCharged()
method. We should, therefore, be able to use the !
symbol, which is a Unary Operator that inverts the value of a boolean, inside our condition along with the isFullyCharged()
method.
Then we just need to charge the battery while it isn't charged. That would go in the do something category. we can accomplish that with the ++
operator and use that on mBarsCount
.
Hopefully that get's you pointed in the correct direction. Post back if you still have questions.
Happy coding,
Ken
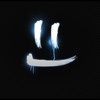
Grigorij Schleifer
10,365 PointsTry it this way ....
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}