Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial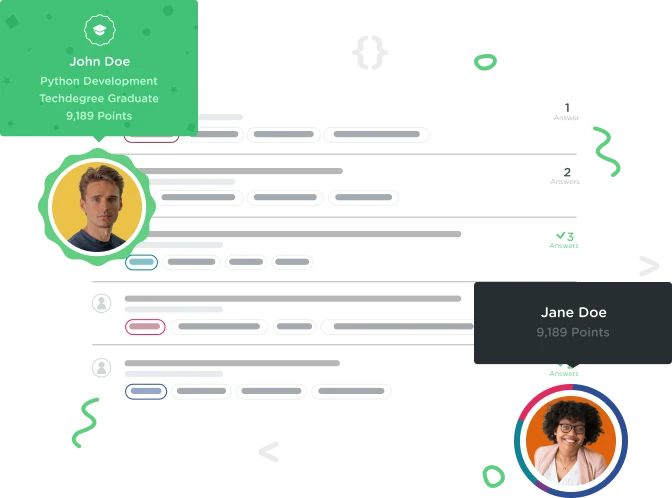

Josep Bordes
7,752 PointsI don't know how to use the property characters. I m using String(string.characters.first).
I d'on't know how to get the first character with the property of string, anyone could help me?
// Enter your code below
extension String {
func modify(a: String -> String) -> String {
return a(self)
}
func firstName(a: String) -> String {
return String(a.characters.first)
}
}
1 Answer
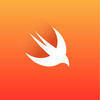
Steven Deutsch
21,046 PointsHey Josep Bordes,
You're on the right track. This challenge can be a bit difficult to wrap your head around, so let's break it down step by step.
First, we need to create an extension on the String type. Inside of this extension, we will create a method called modify. This modify method will be a higher order function. This means that this method will take another function as its argument, with the signature of String -> String. The modify method will also return a String type itself.
Inside the body of the method, we will return the result of the call to the function being passed in. Since this function argument has a return type of String, the value will satisfy the return type of the modify method.
Next, we need to create our function that we are going to pass into the modify method. This will be created outside of the String extension. The method will be called firstLetter, and have a signature of String -> String. It needs to have this signature so that it can match the argument required by the modify method.
Inside the body of this firstLetter method, we will return the first character of the string value we passed in, however, not as a character but as a String.
We can do this by accessing the characters property on the String value being passed in to the firstLetter method. This returns an array of characters for that string. We then want the first character (the first value in the array), we can access this using the .first property which returns an optional character.
Since the .first property returns an optional character, we have to force unwrap it. It's not my favorite thing to forcefully unwrap things, but for the sake of this challenge and because the challenge wants our method to have a non optional String return type, we will do that here.
Finally, we convert the first character in the string's character array back to a String before returning the value.
All thats left to do now is to call the modify higher order function on a string value and pass it in the function that we want it to use.
// Enter your code below
extension String {
func modify(function: String -> String) -> String {
return function(self)
}
}
func firstLetter(value: String) -> String {
return String(value.characters.first!)
}
let value = "Swift".modify(firstLetter)
Good Luck