Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial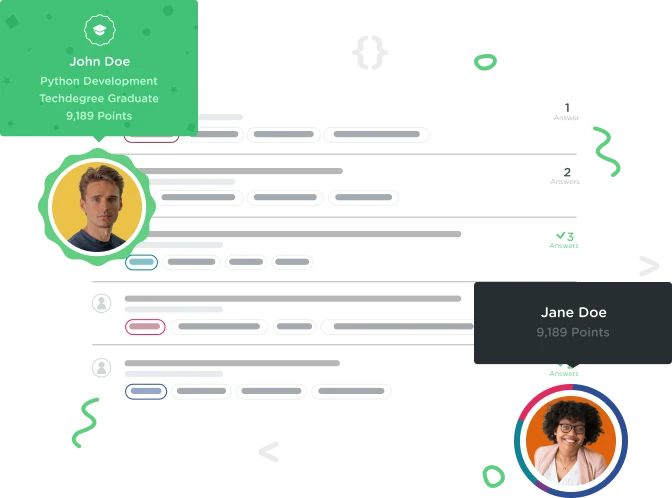

Jacob Headley Porter
2,571 PointsI don't know what i am doing wrong, and i keep going back over everything that I am supposed to use in this part.
I still can't solve it, help with what I did wrong, or I am missing would be greatly appreciated.
function max(upper, lower) {
var upper = 10;
var lower = 2;
if (upper > lower){
}
return upper
}
3 Answers
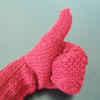
Jonathan Mitten
Courses Plus Student 11,197 PointsReally, you should be naming your arguments neutral names, not upper, not lower - because the tests that get run against this script will mix the order up.
Instead, try
function max(num1, num2) {
if (num1 >= num2){
return num1;
}else{
return num2;
}
}
You also don't want to overwrite your values inside the function. The user (or external code) should be able to set any value to the two arguments and keep them intact. So, remove your first two lines inside the function that over-write the values passed into the function.
Note: It could be said that my code above could be refactored to be even shorter, but I think it's easy to read. However, you should know how return
works. Once the function gets to a return
statement, the function will stop running, having done its job. So:
function max(num1, num2) {
if (num1 >= num2) {
return num1;
}
return num2;
}
will also work. This works because the conditional statement will either return num1
or it'll jump back outside the condition to return num2
. It saves you an else
and a couple lines, but it may not be as obvious to a new coder what is happening.
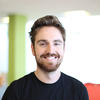
Kristian Woods
23,414 PointsYou haven't set the return inside the if statement
function max(upper, lower) {
var upper = 10;
var lower = 2;
if (upper > lower){
return upper;
}
}

Gari Merrifield
9,598 PointsUsually a max() function takes two or more input values, and returns one of them. So I would add to Kristian Woods' answer :
function max(upper, lower) {
if (upper > lower){
return upper;
} else {
return lower;
}
}
I am also curious why you are reseting the values after accepting them, is that simply for debugging purposes?
Jonathan Mitten
Courses Plus Student 11,197 PointsJonathan Mitten
Courses Plus Student 11,197 PointsFor one, no matter what the user passes to the
max()
function, you're forcibly resetting the values ofupper
andlower
inside the function. If I were to passmax(7, 15)
, your function would replace my7
with10
, and my15
with2
. Secondly, you are naming your input arguments as if you already knew what values might be passed into it. As with my example above, my lowest number is 7, but it's in your argument position asupper
. Instead of using argument names that try to predict the input, use generic names, likenum1
andnum2
. The third reason it's not working as you might expect is because your conditional statement has nothing inside it. Basically it says "if upper is greater than lower, do nothing", because nothing is inside the curly braces. In this case, the function will always return 10, but not becauseupper > lower
. It's because the only action item in the function is toreturn upper
, which unconditional the way you have it written. Move the return statement into the conditional statement if you want the condition to be honored. You'll also want an else. You'll also want to remove thevar upper
andvar lower
variable settings. See my answer below.