Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial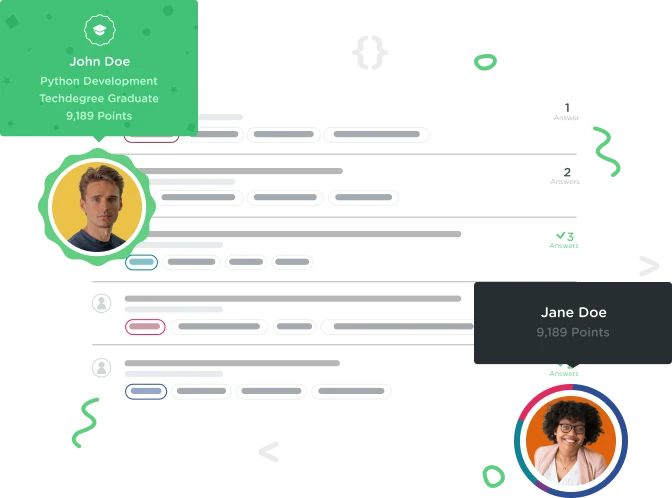

dylan hamm
3,667 PointsI don't know what I did wrong but I keep getting the error "wrong number of prints"
I keep getting the same error. i have no idea what i did wrong
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start == True:
random_number = random.randint(1.99)
number = even_odd(random_number)
if number == 0:
print("{} is even").format(random_number)
else:
print("{} is odd").format(random_number)
start - 1
1 Answer

Umesh Ravji
42,386 PointsHi Dylan, you have a few minor issues in your code.
1. While Condition
The variable start
is a number, so it doesn't really make sense to check to see if it is equal to True
otherwise the while
loop will never run. Probably best to have the condition being start > 0
, but there are other ways.
while start > 0:
2. Generating Random Integer
Probably just a typo, but you need a comma between the two numbers, not a dot.
random_number = random.randint(1, 99)
3. Odd or Even Test Condition
The even_odd
method returns True
(if the number is even) or False
(if the number is odd). You are storing this Boolean value in a variable called number
, which can be a little misleading (maybe you want to rename it). Since A Boolean is either True
or False
the conditional check can be shortened (and comparing it to 0 doesn't make much sense).
if number:
print...
else:
print...
4. Correct Brackets Around Print Statements
The format
method is on the string, not the print
function, so make sure to call it appropriately.
print("{} is even".format(random_number))
5. Decrementing Start
Simply subtracting 1
from start
will not change the value of start. You have to reassign the decreased value to start
.
start = start - 1
# or shorthand
start -= 1
Putting everything together
start = 5
while start > 0:
random_number = random.randint(1, 99)
number = even_odd(random_number)
if number:
print("{} is even".format(random_number))
else:
print("{} is odd".format(random_number))
start -= 1