Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial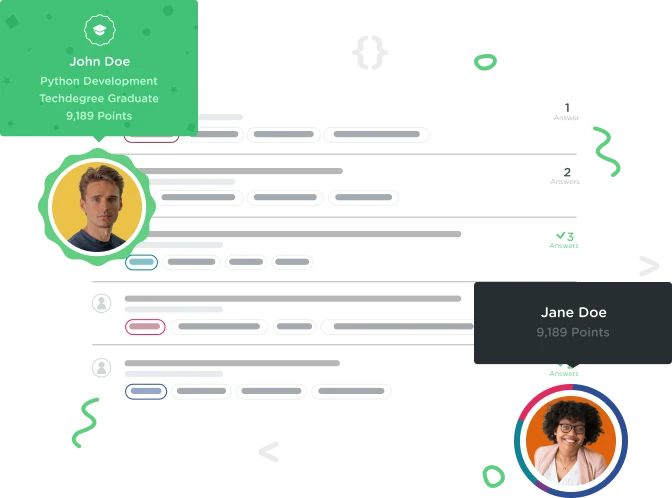
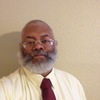
Adiv Abramson
6,919 PointsI don't know what is being asked for here. Where is self.score? How is it populated?
Seems like the score, i.e 5 and 10 is embedded in the string "Player1: 5; Player2: 10". Are we supposed to use place holders in the string for the locations of "5" and "10" and then call format() with the self.score elements as arguments? This is what I wrote:
from game import Game class GameScore(Game): def str(self): self.score = (5, 10) return "Player1: {}; Player2: {}".format(self.score(0), self.score(1))
Where is self.score supposed to be defined? Where do its values come from? Is the task instead to return a tuple (5, 10) and not the string "Player1..."? Is the task to extract "5" and "10" from the string and return a tuple (5, 10)?
I find the instructions very confusing and ambiguous. The error message only says "Try again!" which isn't at all helpful since it doesn't indicate what I'm doing wrong.
from game import Game
class GameScore(Game):
def __str__(self):
self.score = (5, 10)
return "Player1: {}; Player2: {}".format(self.score(0), self.score(1))
8 Answers
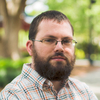
Kenneth Love
Treehouse Guest Teacherself.score
is defined in the Game
class. You don't have to define it (and I didn't tell you to define it for exactly that reason).
Doing "Player 1: {}; Player 2: {}".format(self.score, self.score)
will cause the self.score
tuple to be inserted into your string twice and it won't pass (it did before now, I fixed it so it won't any more because it shouldn't).
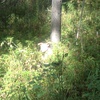
Fareeza Khurshed
1,880 PointsI'm also confused about this question. Can we assume there are only 2 players?
I tried "hard coding" it as follows which won't pass.
from game import Game
class GameScore(Game):
def __str__(self):
return "Player {}: {}; Player {}: {}"
.format(self.score[0][0], self.score[0][1], self.score[1][0], self.score[1][1])
The other option I thought of was using the * argument to unpack, but this also fails.
from game import Game
class GameScore(Game):
def __str__(self):
return "Player {}: {}; Player {}: {}"
.format(*self.score[0], *self.score[1])
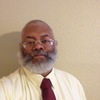
Adiv Abramson
6,919 PointsIn your code you have
Python
def str(self):
I think you need to write
Python
def __str__(self):
which is the built in method that we're supposed to override. I think when you use
Python
print <some_object>
Python calls the str method of <some_object> .
When we explicitly define a str method in a class definition
we're coding, that replaces (overrides) the built-in str that every class contains.
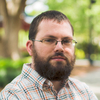
Kenneth Love
Treehouse Guest TeacherIt doesn't really matter if there are more than 2 players or not, since you'll only be getting updates for either player 1 or player 2. But, remember, you'll have to adjust which player gets updated (if you get 1
, you'll want to change self.score[0]
.) Your self.score
attribute is just a single list, so you can't do self.score[0][0]
, for example.
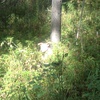
Fareeza Khurshed
1,880 PointsBlah, the forum ate the underscores, in your post as well :)
I figured it out anyways, used unpacking of tuples instead, works like a charm.
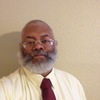
Adiv Abramson
6,919 PointsI just now noticed that. I think if you enclose a code block in triple backticks (```) then the formatting is someone preserved. There should be a standard HTML <pre> tag that we could use when we wish to include code in our comments.
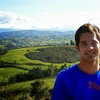
Dhruv Ghulati
1,582 PointsThanks!! And I made the silliest error - my string had spaces in the wrong places. Appreciate your responsiveness.
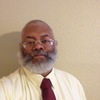
Adiv Abramson
6,919 PointsThank you. I tried the exercise again earlier today. I just returned the "Player1: 5; Player2: 10" literal and it was accepted.
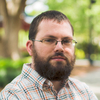
Kenneth Love
Treehouse Guest TeacherYep, that was also a bad solution that was allowed. It shouldn't be any more.
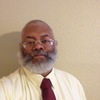
Adiv Abramson
6,919 PointsWas the objective to return a tuple, like so: return (5, 10) or was it to return a string using the format function to provide values (from the self.score object, defined in the parent class) to replace the {} placeholders embedded in said string or was something else required? I am sorry but I haven't yet been able to determine what the requirements are for this brief exercise.
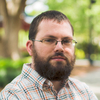
Kenneth Love
Treehouse Guest TeacherYou're returning a string. Overriding the __str__
method changes how a class instance becomes a string. The video before this was about exactly that.

Scott Dunstan
12,517 PointsWow, totally forgot about unpacking tuples with a ' * '... got stuck for ages.
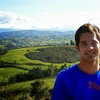
Dhruv Ghulati
1,582 PointsHi,
I am stuck here also. I have:
import game
from game import Game
class GameScore(Game):
pass
def __str__(string):
return ('Player {}: {}; Player {}: {}'.format('1',*self.score[0],'2', *self.score[1])
Not sure what I am doing wrong as I am making both the player number and score variable, however I know that there is only 1 self argument. How do I unpack the tuple into the return string?
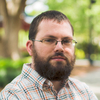
Kenneth Love
Treehouse Guest Teacher__str__
needs to take self
, not string
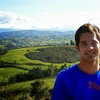
Dhruv Ghulati
1,582 PointsOK, then do I need to replace '1' by *self[0] and '2' by *self[1]?
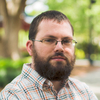
Kenneth Love
Treehouse Guest TeacherIf you're going to use indexes, you don't need the asterisks. And you'll need to always reference self.score
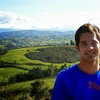
Dhruv Ghulati
1,582 PointsWhat I don't understand is what in self.score represents the player - I guess it is the index number of a tuple? stack overflow wasn't very helpful in this.
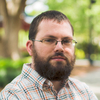
Kenneth Love
Treehouse Guest TeacherSince self.score
has an order, yes, you can assume that the first position is the first player, second position is the second player, and so on (if we had more than 2 players).
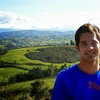
Dhruv Ghulati
1,582 PointsMy question then becomes - how do I use format to fill in "1" and "2" given I have to use self.score as a variable? Or did I have to even do that? I did it because of a comment you made earlier:
*But, remember, you'll have to adjust which player gets updated (if you get 1, you'll want to change self.score[0].) *
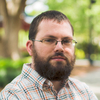
Kenneth Love
Treehouse Guest TeacherYou can just use "Player 1: {}; Player 2: {}"
. The player numbers aren't going to change.