Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial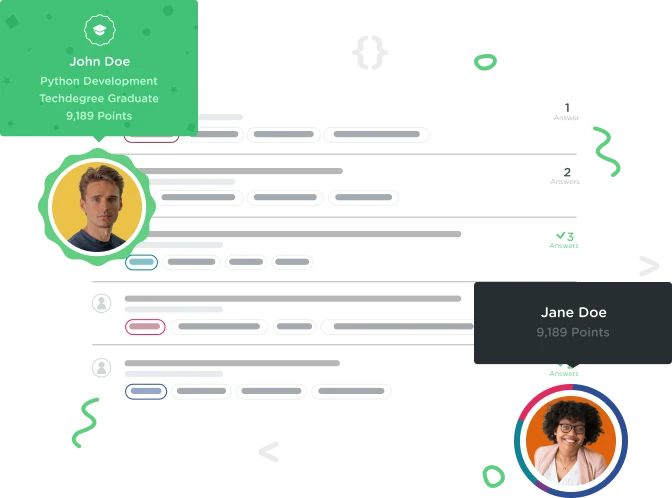
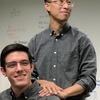
Robert Hutchins
4,257 PointsI don't know what is wrong with my code in this exercise. I have made a constructor how the task requested.
I am also having issues with the workspaces I followed the video to the t and am getting an error when trying to compile.
Game.cs(7,21): error CS0122: `TreehouseDefense.Map.Map(int, int)' is inaccessible due to its protection level
Map.cs(9,8): (Location of the symbol related to previous error)
Compilation failed: 1 error(s), 0 warnings
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
Frog(int tongueLength)
{
int TongueLength = tongueLength;
}
}
}
1 Answer

andren
28,558 PointsThere are two errors, the first is that you don't specify an access level (public, private, etc) for the constructor, constructors (in most instances) have to be public, but if you don't specify an access level explicitly then it will default to private.
The second issue is that inside of the constructor you are meant to set the TongueLength variable that already exists as a member variable in the class to the value that is passed in to the constructor.
In your solution you are not setting the existing TongueLength to a new value, you are creating a new TongueLength variable within the constructor and assigning a value to that new variable. The reason for that is that you specify the data type of the variable, that is only done when you want to create a variable for the first time, when modyfing an existing variable you are not meant to specify it's data type.
Fixing those two issues results in this code:
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int tongueLength) // Added public to constructor declaration
{
TongueLength = tongueLength; // removed int data type
}
}
}
Which will pass the first task of the challenge.
Also as for the error you are getting in the workspace, while it's impossible to say for sure without looking at the code, my guess would be that you forgot to set the access level of the Map constructor to public just like you did with this task. The error message is essentially telling you that you are trying to access a constructor whose access level is not set to a value that would allow access from the place where you are calling it from.