Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial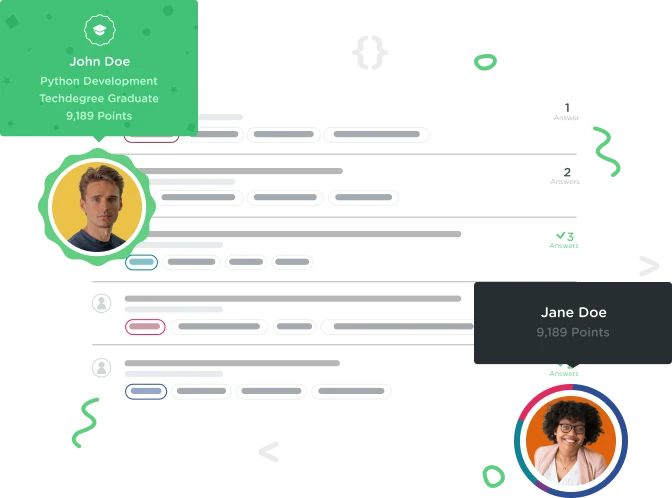
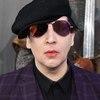
Adham Ali
Courses Plus Student 1,112 PointsI dont know what is wrong with my code. It throws a NullArgumentException
I did as it told me... if the user entered a invalid input it catches It and recall the method instead of including the whole method body in a loop and writing continue to jump to the top of the code
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string userInput = Console.ReadLine();
var numbers = 0;
try
{
numbers = int.Parse(userInput);
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
Main();
}
int counter=0;
while(numbers != counter)
{
Console.WriteLine("Yay!");
counter++;
}
}
}
}
4 Answers
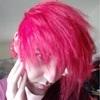
Patrik Horváth
11,110 PointsTASK 1 : you need FOR LOOP
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string userInput = Console.ReadLine();
for (int i=0; i < int.Parse(userInput); i++)
{
Console.Write("Yay!");
}
}
}
}
TASK 2:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string userInput = Console.ReadLine();
try {
for (int i=0; i < int.Parse(userInput); i++)
{
Console.Write("Yay!");
}
} catch (Exception e) {
Console.Write("You must enter a whole number.");
}
}
}
}
TASK 3 :
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string userInput = Console.ReadLine();
try {
if (int.Parse(userInput) < 0) {
Console.Write("You must enter a positive number");
} else {
for (int i=0; i < int.Parse(userInput); i++)
{
Console.Write("Yay!");
}
}
}catch (Exception e) {
Console.Write("You must enter a whole number.");
}
}
}
}
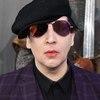
Adham Ali
Courses Plus Student 1,112 Pointsyeah but whats wrong with my code?
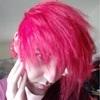
Patrik Horváth
11,110 Pointsfirst part was good but why number = 0 ? you can simple
int number = numbers = int.Parse(userInput);
then inside TRY you have to loop Console.Write based on user INPUT ( number )
and you CANT call main() because its not allowed in any programing language call MAIN method / function, because main can be called only ONCE when probgram RUNs
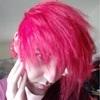
Patrik Horváth
11,110 Pointsif you want LOOP something more times you have to use FOR / WHILE / DO WHILE / FOR EACH
if not your program will not repeat
try on your own write numbers from 0 to 100 :)
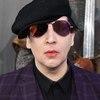
Adham Ali
Courses Plus Student 1,112 PointsFirst of all: I always call Main Method (BUT INSIDE THE BODY OF ITS OWN).
Because simply its just a method. Yeah the compiler runs it in the beginning, It
s the entry point.
Maybe it uses reflection or something I wish I knew.
But it is just a method. How it could prevent us from calling it
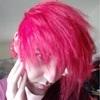
Patrik Horváth
11,110 Pointsyea you can call main() because its only 1 way how to run program :)
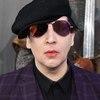
Adham Ali
Courses Plus Student 1,112 PointsI run it maunaly in the code I write for instance for jumping the the beginning of the method if some input was invalid and I print a text to make the user enter an input again