Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial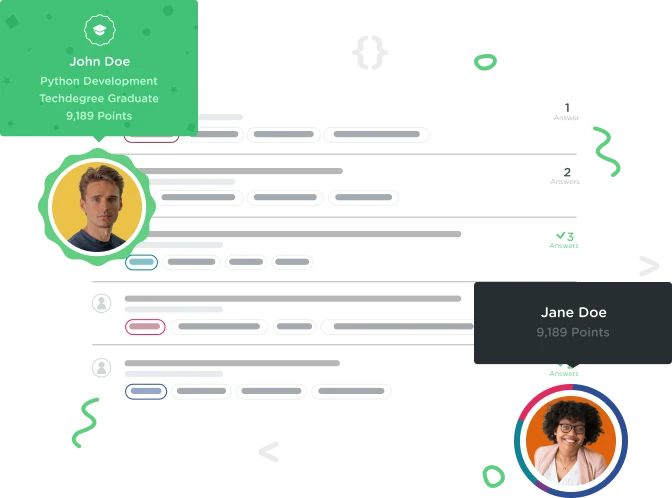

Eduardo Cassinelli
1,546 PointsI dont know what is wrong with my code of the catch exception, pls help
The error seems to be in the catch line, I keep getting the reminder of adding a catch block for the exception, the example from the video is quite different from the one in the task I thing I'm doing sth wrong.
int value = int.Parse(Console.ReadLine());
if (value < 0 || value > 20)
{
throw new System.Exception();
}
Console.WriteLine(string.Format("You entered {0}",value));
try
{
value = 30;
}
catch (System.Exception)
{
Console.WriteLine("Value is out of bounds!");
}
3 Answers
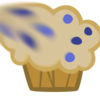
David McCarty
5,214 PointsHere's why!
The bit of code:
if (value < 0 || value > 20)
{
throw new System.Exception();
}
Is what is throwing the error. You need to catch that error if and when it is thrown. Simply setting Value = to something outside of the range 0 - 20, will not throw an error. The if statement is checking to see if the value is greater than 20 or less than 0. IF it IS, then it is throwing the System.Exception() exception. What you need to do is wrap a try/catch block around where said exception is being Thrown from.
try
{
if (value < 0 || value > 20)
{
throw new System.Exception();
}
}
catch (System.Exception ex)
{
Console.WriteLine("The value is out of bounds!");
}
That should do it!
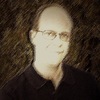
Jason Anders
Treehouse Moderator 145,860 PointsHi Eduardo,
I feel you may be overcomplicating the task. Basically all you need to do for this task is encapsulate the give code in the try/catch block.
The challenge didn't ask you to declare any values, so that line needs to be deleted first off. Next, you need to move the try
block up to surround the if statement
. If the if statement returns false (in bounds) it will jump and execute the Console.WriteLine to tell which value was entered.
If the if statements
returns true (out of bounds), it will throw the error and jump to the catch block
and return the line that states you are out of bounds.
Below is the corrected code for your reference. I hope it'll make sense. :)
int value = int.Parse(Console.ReadLine());
try {
if (value < 0 || value > 20)
{
throw new System.Exception();
}
Console.WriteLine(string.Format("You entered {0}",value));
} catch {
Console.WriteLine("Value is out of bounds!");
}

Eduardo Cassinelli
1,546 PointsThat was really helpful thx.
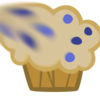
David McCarty
5,214 PointsHappy to help!
Feel free to mark the answer that helped you the most as "Best Answer" to help future users find the answer to such a problem