Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial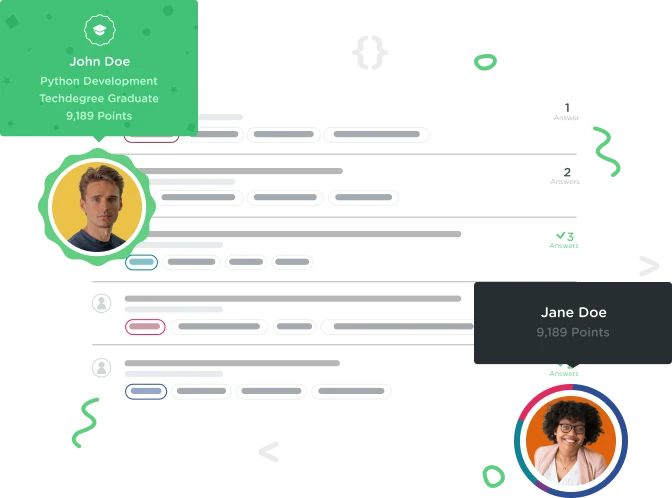

ISAIAH S
1,409 PointsI don't know what this challenge is asking for.
We are currently in the process of building a Forum. There is some skeleton code here, but I need your help to finish it up. Let's do this!
In the Forum class, add a constructor that takes a String for the topic and sets the private field mTopic.
Important: the code you write in each task should be added to the code written in the previous task.
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
public User(String firstName, String lastName) {
// TODO: Set the private fields here
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public void addPost(ForumPost post) {
/*When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
// Forum forum = new Forum("Java");
// Take the first two elements passed args
// User author = new User();
// Add the author, title and description
// ForumPost post = new ForumPost();
// forum.addPost(post);
}
}
3 Answers
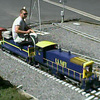
Stephen Goodwin
5,479 PointsRead up on what a constructor is here > http://www.homeandlearn.co.uk/java/class_constructor.html

Raymond Wach
7,961 PointsHi ISAIAH S, to complete this challenge, you only need to look at Forum.java because that is where the Forum class is defined. The challenge is asking you to add a constructor to the Forum class; this means that you need to implement a Forum constructor that takes a String argument and uses that value to set the mTopic
(private) instance variable.
You can see that mTopic
is declared as a private instance member of type String
on the first line of the body of the Forum class, but it is never initialized; that will be done in the constructor you are about to write. The syntax for declaring a constructor in Java is as follows:
// <visibility> <type> <parameter list>
public Example (String arg0, int arg1, Object arg2) {
// Body of the constructor
}
Most of the time, we want our constructor to be public so that it can be called from outside of the current class. Inside our constructor, we have access to private members of the class so this is where we can initialize those members (mTopic
, in your case) by assigning them values for the first time. Typically, the value that we want is declared as a parameter of the constructor so we often see something like:
class Foo {
private String myFoo;
private String myBar;
public Foo (String foo, String bar) {
myFoo = foo;
myBar = bar;
}
}
This makes sense because the constructor is there to guarantee the safe and successful construction of new instances of the class. In order to get a new instance of a class, other code will call your class's constructor with whatever arguments (or parameters, they mean about the same thing) are required to successfully instantiate your class.

ISAIAH S
1,409 PointsI still don't know how to complete the challenge.

Raymond Wach
7,961 PointsTo complete the challenge you just need to modify Forum.java
, the Forum class, by adding a constructor (a type of method) that sets mTopic
. Your constructor will need to take a String
argument to determine what value to use to set mTopic
.

ISAIAH S
1,409 PointsI pressed Check Work after I wrote:
public class Forum {
private String mTopic;
public setTopic(String getTopic) {
mTopic = getTopic;
}
public String getTopic() {
return mTopic;
}
public void addPost(ForumPost post) {
/* When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
and I get this error:
./Forum.java:4: error: invalid method declaration; return type required
public setTopic(String getTopic) {
^
1 error
thanks!!

Raymond Wach
7,961 PointsYou're making progress, but what you have written looks more like a method declaration (and implementation) than a constructor. A method declaration differs from a constructor in that it has a name and a return type. These things are implicit with the constructor: they are the class to be constructed. You need to make your method have the name of class for which the method will construct objects.

Jess Sanders
12,086 Points public setTopic(String getTopic) {
mTopic = getTopic;
}
This is so close!!! But, a constructor should have the same name as the class (In this case "Forum"). Change that, and you've got it!
ISAIAH S
1,409 PointsISAIAH S
1,409 PointsThanks Stephen!!!