Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial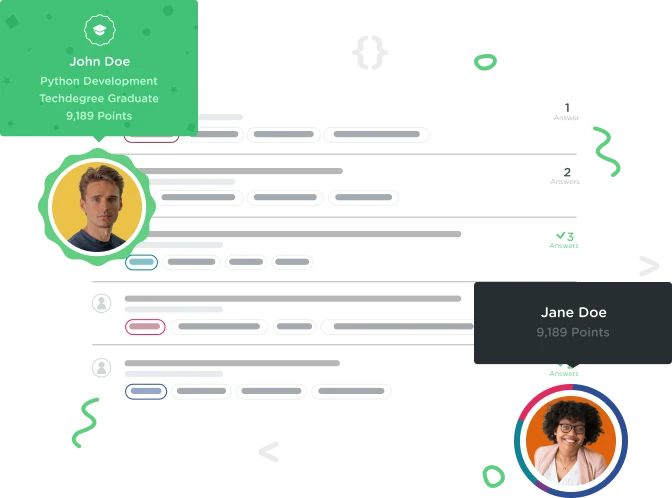

Justus Aberson
1,035 PointsI don't know what to do next
Hey guys, I did the first part of the question but I don't know what to do as 2nd part. I've tried to creat an instance in Xcode to try, but it didn't work out. I hope anyone can help me what to do.
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = Location.init(latitude: 0.00 , longitude: 0.00)
}
}
1 Answer
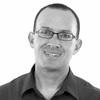
David Papandrew
8,386 PointsHi Justus,
Here is the corrected code, I will try to explain below:
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = location
}
}
let someBusiness = Business(name: "Store XYZ", location: Location(latitude: 10, longitude: 20))
To call the class properties you can do this:
someBusiness.name
someBusiness.location.latitude
someBusiness.location.longitude
Main issue in your code was that you were trying to call Location init in the Business init method. You don't need to do this. Remember, you get a "free" initializer for structs (notice how your struct has no init method but you can still type something like: let someLocation = Location(latitude: 100, longitude: 100) and you can create a new Location instance.
All you need to do is make sure the Business class location property is taking the Location argument being passed in when the Location init is called. (this is the "self.location = location" line.
Hope that helps.