Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial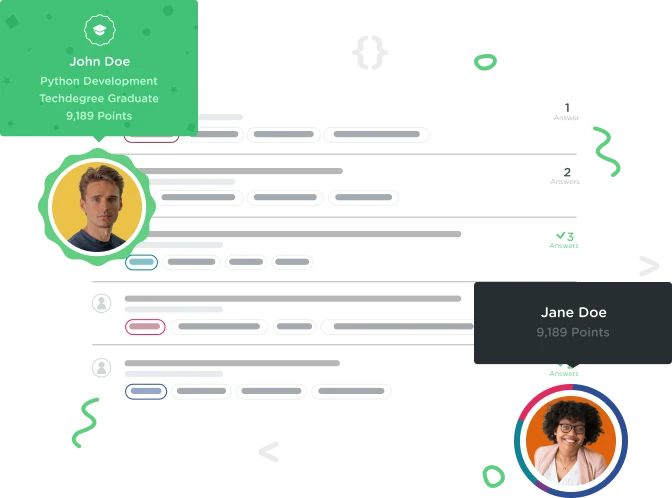

Donald Beaudin
4,461 PointsI don't know what to do now?
If some one could help that would be Great!!! Here's the '?' Challenge Task 1 of 2
"Inside the onCreate() method (after the setContentView() call), log the message "Activity created!" using the Log debug method (remember that it's just one letter). The 1st parameter should be "TreehouseActivity", but use the TAG variable instead of typing it out a second time. The 2nd parameter is the message."
package com.teamtreehouse;
public class TreehouseActivity extends Activity {
public static final String TAG = "TreehouseActivity";
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_treehouse);
}
}
3 Answers
Rebecca Rich
Courses Plus Student 8,592 PointsAndroid has a built in API for logging outputs -- Log
. Log has many different flavors, one of which is for debugging (you can filter your logs by these flavors). Logs have a tag and a message associated with them. The tag is usually used to identify the class or activity the log is located in and the message is a more verbose description of whatever it is you want to log.
In this case, we want the tag to be "TreehouseActivity" represented by the static String TAG
. We want the message to be "Activity created!":
Log.d(TAG, "Activity created!");
or within the onCreate method:
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_treehouse);
Log.d(TAG, "Activity created!");
}
Hope this helps!
Here is a link with some more info about debug tags and other tag types: http://developer.android.com/reference/android/util/Log.html
Rebecca Rich
Courses Plus Student 8,592 PointsHi Immanuel,
The msg: portion is a lighter gray color. It is meant to be a hint or reminder from the IDE (in this case Android Studio) as to what the second argument of the method being called is. Notice in the documentation that the second argument for the Log.d is a String referred to as msg. You will see a similar hint for the tag.
βmsg:β is not actually typed in the code and itβs visibility should be able to be controlled by the IDE settings.
Does this help?

Immanuel Tan
2,214 PointsYes, thank you very much!! :D
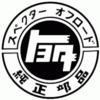
mongst
10,841 PointsSpoiler Alert:
package com.teamtreehouse;
public class TreehouseActivity extends Activity {
public static final String TAG = "TreehouseActivity";
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_treehouse);
Log.d(TAG,"Activity created!");
}
}
Immanuel Tan
2,214 PointsImmanuel Tan
2,214 PointsHi, Rebecca, why is the answer, not Log.d(TAG, msg: "Activity created!"); as shown in the video. I'm not sure why the msg: was taken out?