Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial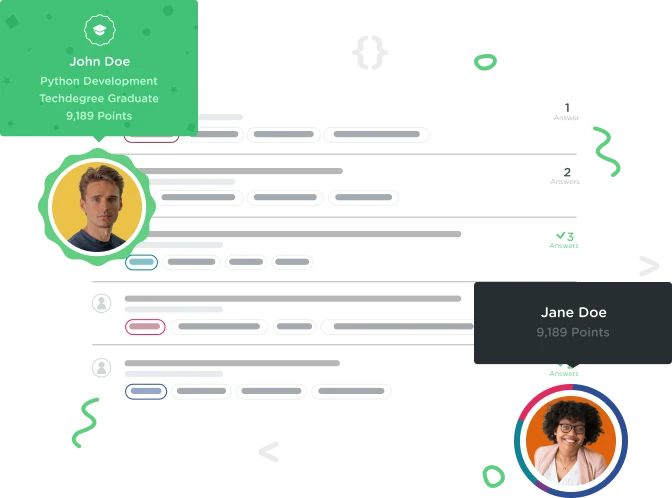

nuri jeon
14,376 PointsI don't know where to pass the arguments.(Between __init__ or super()__init__())
import random
class Die:
def __init__(self, sides=2, value=0):
if not sides >=2:
raise ValueError("Must have at least 2 sides")
if not isinstance(value, int):
raise ValueError("sides must be a whole number")
self.value = value or random.randint(1, sides)
class D6(Die):
def __init__(self, value=0):
super().__init__(sides=6, value=value)
In D6's init method, I think it should work same way if I put them these ways too.
def __init__(self, sides=6, value=0):
super().__init__(sides=sides, value=value)
def __init__(self, sides=6):
super().__init__(sides=sides, value=0)
I'm not sure what kind of difference does this make :O... So the question is@@ I'm not sure where to pass my arguments...
[MOD: added ```python formatting -cf]
1 Answer
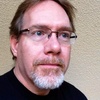
Chris Freeman
Treehouse Moderator 68,423 PointsGreat question! You are correct that the instance created in all three cases would be the same if only the default values were used. Let's look at what happens when non-default arguments are present.
In the original case of D6.__init__(value=0)
, the number of sides is hardcoded to be six in the super call since the class is supposed to be for a six-sided die. Only the initial value can be specified. Note that since value
is the only parameter, it can be specified as a positional or a keyword argument.
In the second case of D6._init__(sides=6, value=0)
, the ability to specify the number of sides is introduced which makes the class D6
basically the same as the class Die
. This leaves the D6 __init__
method without any added value and the method could be removed. Without an overridden __init__
, the D6 class is no different than its inherited Die class. Also, by adding the sides parameter, specifying a value argument can now only be done using a keyword argument.
In the third case of D6.__init__(sides=6)
, the sides can be specified, which goes against the point of a D6 class, and the ability of specifying an initial value is lost.
So while the cases seem equivalent in the default cases, the practical use of the parameters yields undesirable side effects in the alternative definitions.
Post back if you have more questions. Good luck!!!
nuri jeon
14,376 Pointsnuri jeon
14,376 PointsAh.. I think I completely forgot about the point of using inheritance at that moment XD.... Your explanation helped me realised it very clearly! Thank you very much sir :D