Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial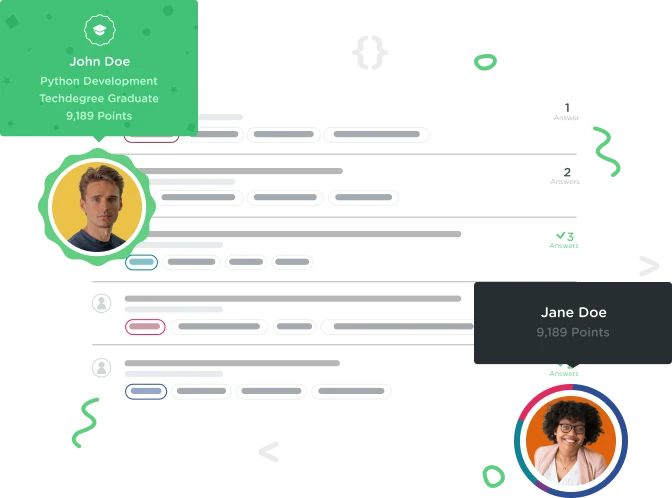

Phillip Rowe
Courses Plus Student 13,741 PointsI don't know why "Bummer! Didn't get the right string output. Got: dot-dot-dot for `S()`" is an error.
Bummer! Didn't get the right string output. Got: dot-dot-dot for S()
class Letter: def init(self, pattern=None): self.pattern = pattern
class S(Letter): def init(self): pattern = ['.', '.', '.'] super().init(pattern)
def __str__(self):
morse=[]
for item in self.pattern:
if item==".":
morse.append("dot")
morse.append("-")
else:
morse.append("dash")
morse.append("-")
morse=morse[:-1]
return "".join(morse)
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
def __str__(self):
morse=[]
for item in self.pattern:
if item==".":
morse.append("dot")
morse.append("-")
else:
morse.append("dash")
morse.append("-")
morse=morse[:-1]
#print(''.join(morse))
return "".join(morse)
1 Answer

andren
28,558 PointsThat message is a bit misleading. The issue with your code is that the __str__
method is supposed to be defined in the Letter
class, not the S
class which inherits from Letter
.
Like this:
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
morse=[]
for item in self.pattern:
if item==".":
morse.append("dot")
morse.append("-")
else:
morse.append("dash")
morse.append("-")
morse=morse[:-1]
#print(''.join(morse))
return "".join(morse)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
Also just as a note I would like to point out that instead of adding the "-" character to the list and then slicing it at the end, you could instead just use "-" as the joining string.
Like this:
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
morse=[]
for item in self.pattern:
if item==".":
morse.append("dot")
else:
morse.append("dash")
return "-".join(morse) # Join the list with a - between the items.
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
That is a bit cleaner and more efficient.