Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial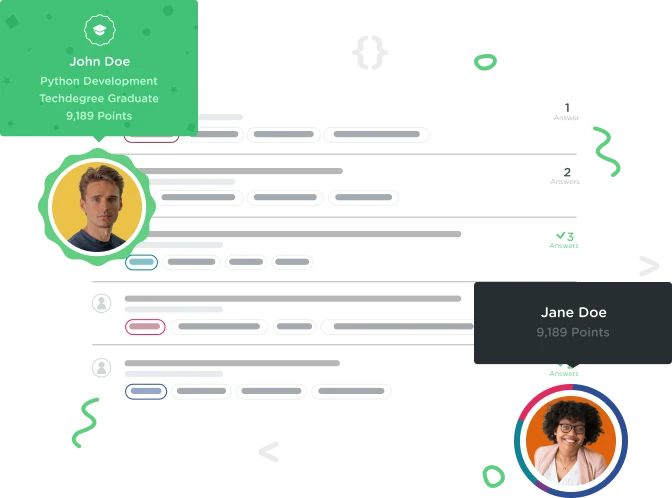

Hana Tran
3,897 PointsI dont know why I cant pass task 2
Can someone help me? Or it's a Treehouse bug?
var objects = [
{food: cake, drink: milk shake},
{food: pizza, drink: cola},
{food: salad, drink: water}
];
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Objects</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
4 Answers

Christopher McRoy
17,295 PointsLooks like the problem is you're missing quotes around your values. The quotes are necessary here because your values are strings.
So, your code should look like this:
var objects = [
{food: 'cake', drink: 'milk shake'},
{food: 'pizza', drink: 'cola'},
{food: 'salad', drink: 'water'}
];
Keep up the great work!
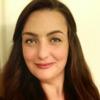
Jennifer Nordell
Treehouse TeacherHi Hana! First, you're doing great! And this would pass if it weren't for one little thing. All the things on the right sides of the colon :
should be string literals. This means they should be surrounded in quotes. If you don't do this, JavaScript believes them to be variables. This is the case right now. So if you start by putting quotes around the things on the right side of food:
and drink:
, your code passes!
Hope this helps!

Mike Wagner
23,559 PointsI was just about to say that but it took me a few to figure it out, haha. It would be helpful if the error it provided in the challenge was helpful. It was saying that task 1 was no longer passing, which was misleading. I would think, in this case, it should have said something about undefined variables, or at least something slightly more appropriate and related to task 2 not meeting completion.
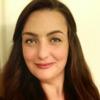
Jennifer Nordell
Treehouse Teachermwagner I know that the Bummer! messages can be difficult to interpret on occasion. But, generally speaking, when you see a "Task <insert number> is no longer passing", it means that there's been a syntax error introduced into the code, so that it can't even be run properly. There are other causes as well, but this is the primary one. And that was the case here. The missing quotes made JavaScript believe the coder was referencing undefined variables.

Mike Wagner
23,559 PointsJennifer Nordell - I understand that, but it is far from intuitive. Some of the challenges actually do a pretty decent job of providing more detailed messages, and I've even had a few mention syntax issues, but in this case, a blatant syntax error wasn't the issue, it was the use of undefined variables. Since this is one of so many challenges that doesn't (for some reason) provide access to a "preview" button to see the 'console' output, it leaves the problems unavoidably obscure without any real way to debug the problem. Being a seasoned Treehouse user, I myself understand that sometimes those "Bummer!" messages are a bit misleading, but it doesn't help the user experience any, especially with people less familiar with Treehouse challenge quirks.

Christopher McRoy
17,295 Points"I understand that, but it is far from intuitive. Some of the challenges actually do a pretty decent job of providing more detailed messages, and I've even had a few mention syntax issues, but in this case, a blatant syntax error wasn't the issue, it was the use of undefined variables." -mwagner
I think one of the main reasons for less-than-helpful error messages is that the errors have to be predetermined and programmed in as possibilities. I think a teacher can expect and plan for a couple of likely errors, but I don't know if they can really plan for "typos", which is pretty much what this issue is (even though the typo resulted in a lot of undefined variables). In a way, the error message is trying to tell you that you forgot to define a variable by saying "task 1 is no longer passing", because it thinks there should be only one variable (named 'objects'), which was defined properly in task 1. Now that it sees an undefined variable, it thinks you must have removed 'var' from 'objects', making it undefined.
I feel like I'm not explaining it well, so here is what I'm trying to say in code examples.
Task 1 asks you to create an array literal, which requires the 'var' keyword. A new coder might forget this, and write this instead:
objects = [];
The teacher did plan for this, and if you do forget 'var', you will receive this error message: "Bummer! Make sure you declare the objects
array with the var
keyword."
Task 2 asks you to fill the array literal with property/value pairs, but doesn't ask for any additional variables. So if you passed Task 1 but then added an undefined variable in Task 2, the program thinks you changed the code you wrote in Task 1.
//Passed Task 1 with:
var objects = [];
//Then in Task 2, changed original code to something like this:
objects = [
{food: 'cake', drink: 'milk shake'},
{food: 'pizza', drink: 'cola'},
{food: 'salad', drink: 'water'}
];
"Since this is one of so many challenges that doesn't (for some reason) provide access to a "preview" button to see the 'console' output, it leaves the problems unavoidably obscure without any real way to debug the problem. Being a seasoned Treehouse user, I myself understand that sometimes those "Bummer!" messages are a bit misleading, but it doesn't help the user experience any, especially with people less familiar with Treehouse challenge quirks." -mwagner
It's true, it does make debugging difficult without a "preview" option. Being resourceful is a major part of coding, though, and I believe any challenge that requires thinking outside the box is going to be helpful. In the real world, we will run into problems with our code, and the resulting error messages are likely to be just as obscure and misleading (if not more so).
I found the best way around the lack of a "preview" option is to copy all of the HTML and JS code into my Notepad++ program, save the files on my computer, run them in Chrome and then debug using the console that way. This may sound like a lot of work, but it's actually great experience for using the console and other external resources.
Hope this is helpful!
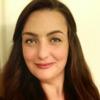
Jennifer Nordell
Treehouse TeacherI agree with Christopher on this. In the real world, there would be nothing to "Preview" even if you could. This code, in and of itself, does nothing to display anything to the webpage. Above and beyond that, even an error wouldn't print to the webpage. The only option to debug this would be launching the console to view errors. I also use the same tactic Christopher does and try and debug my code locally first. I could be wrong here, but I believe part of the problem lies in that this is not a compiled language. There will be no compilation errors ... only things that the browser can't interpret. And if the browser can't interpret them, then nothing will be available to view on the site.

Mike Wagner
23,559 PointsI think one of the main reasons for less-than-helpful error messages is that the errors have to be predetermined and programmed in as possibilities. I think a teacher can expect and plan for a couple of likely errors, but I don't know if they can really plan for "typos", which is pretty much what this issue is." - Christopher McRoy
Christopher McRoy - I would agree with this, and generally do, aside from the fact that this isn't an error that needs to be "planned" for. Using this code the way it sits, results in a built-in "Reference Error" which the Challenge would actually show if it had the "preview" button to debug. This isn't something the instructor needs to account for, it just exists. Some of the Challenges actually do a really go job of returning things like syntax errors and reference errors just fine (even without a preview button to glance at the compiler/interpreter output. This one simply says "Looks like task 1 is no longer passing," which is far from the truth, because you can "go to task one" and hit recheck work and it passes and sends you back to task 2.
Jennifer Nordell - You're wrong when you say there would be nothing to "preview" even if you could. There is always compiler/interpreter output that returns built-in and user-defined errors, the ability to print() or write(), etc. This is what the "preview" button tends to do in challenges that have it but don't actually display a webpage. Again, however, even without the "preview" (or a console, for some reason, but that's another ball of wax) option available, about half of the challenges I've done, in the many courses I've run through, have actually returned the errors or at least helpful output alongside the "Bummer!" message. Others...behave like this.
The only option to debug this would be launching the console to view errors. I also use the same tactic Christopher does and try and debug my code locally first.
And this is true, I agree with it whole-heartedly and practice the same myself, but that isn't what's actually being talked about here. It's the lack of proper messages being returned that make challenges difficult to debug without a secondary console running the language at hand or a Workspaces open alongside challenges (which still give you erroneous "bummer" messages, so you're looking in the wrong place half the time anyway). Considering Treehouse has been trying to promote itself as a "take me anywhere" learning environment with the iOS and Android apps, this simply isn't an option for some that are on the go or use those apps as a primary means to make progress in courses anyway.
I'll leave it at that. There's not much need for a response at this time since it would probably be more of the same stuff. I only intended to shed a bit of light on the fact that the UX as a whole suffers because of something like this. I thoroughly enjoy Treehouse and think that the team does a great job with what it does. I just think in some small ways, it could be better.

Hana Tran
3,897 PointsThank you all so much! I figured it out. Silly me :P And I agree that it would be better if the Bummer is more accurate and specific.