Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial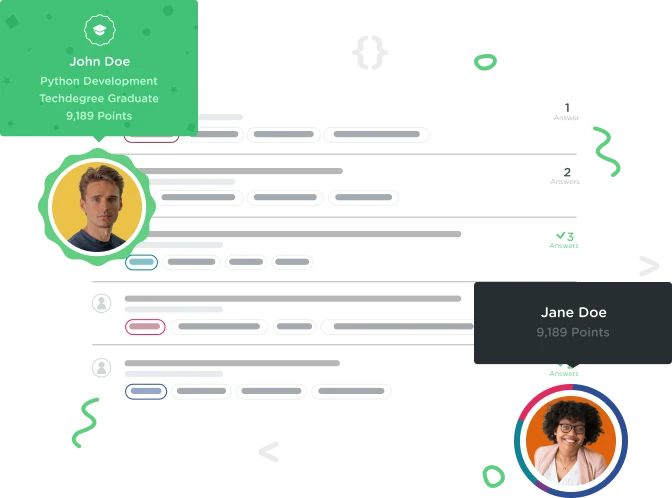

scott twitchell
4,915 Pointsi dont know why my data isnt being saved to the db?
i was fallowing along with the "user authentication with express and mongo" but mongoose was out of data and causing errors. i think i fixed that but no data is being saved to the db.
User.js
const mongoose= require("mongoose");
const Promise = require('promise');
var UserSchema = new mongoose.Schema({
email: {
type: String,
unique: true,
required: true,
trim: true,
},
name: {
type: String,
required: true,
trim: true,
},
password: {
type: String,
required: true
}
});
UserSchema.statics.authenticate = function(email, password, callback) {
User.findOne({ email: email })
.exec(function (error, user) {
if (error) {
return callback(error);
} else if ( !user ) {
var err = new Error('User not found.');
err.status = 401;
return callback(err);
}
bcrypt.compare(password, user.password , function(error, result) {
if (result === true) {
return callback(null, user);
} else {
return callback();
}
})
});
}
// hash password before saving to database
UserSchema.save('save', function(next) {
var user = this;
bcrypt.hash(user.password, 10, function(err, hash) {
if (err) {
return next(err);
}
user.password = hash;
next();
})
});
app.js
var User = mongoose.model('User', UserSchema);
module.exports = User;
const express = require("express");
const passport= require("passport");
const mongoose= require("mongoose");
const bodyParser = require('body-parser');
const Promise = require('promise');
const session = require('express-session');
const app = express();
app.use(session({
secret: 'treehouse love you',
resave: true,
saveUninitialized: false
}));
const models = require('./models/user');
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: false }));
app.use(express.static(__dirname + '/public'));
var MongoClient = require('mongodb').MongoClient
, assert = require('assert');
// Connection URL
var url = 'mongodb://localhost:27017/flash';
// Use connect method to connect to the server
MongoClient.connect(url, function(err, db) {
assert.equal(null, err);
console.log("Connected successfully to server");
db.close();
});
app.set('view engine','pug');
app.set('views', __dirname + '/views');
var routes = require('./routes/index');
app.use('/', routes);
app.get("/",(request,response)=>{
return response.render("layout",{title:'sighn up'})
});
app.get("/home",(request,response)=>{
response.render("home");
});
app.get("/register",(request,response)=>{
response.render("register");
});
app.get("/login",(request,response)=>{
response.render("login");
});
app.get("/profile",(request,response)=>{
response.render("profile");
});
app.use(function(err, req, res, next) {
res.status(err.status || 500);
res.render('error', {
message: err.message,
error: {}
});
});
app.listen(3000);
index.js
var express = require('express');
var router = express.Router();
var User = require('../models/user');
router.get('/login', function(req, res, next){
return res.render('login', {title: "Log in"})
});
router.post('/login', function(req, res, next){
if(req.body.email && req.body.password){
}else{
var err = new Error('Email and password are reqwuired.')
err.status = 401;
return next(err);
}
});
// GET /register
router.get('/register', function(req, res, next) {
return res.render('register', { title: 'Sign Up' });
});
// POST /register
router.post('/register', function(req, res, next) {
if (req.body.email &&
req.body.name &&
req.body.password &&
req.body.confirmPassword) {
return res.send('user created');
if (req.body.password !== req.body.confirmPassword) {
var err = new Error('Passwords do not match.');
err.status = 400;
return next(err);
}
User.create(userData, function (error, user) {
if (error) {
return next(error);
} else {
return res.redirect('/profile');
}
});
} else {
var err = new Error('All fields required.');
err.status = 400;
return next(err);
}
})
// GET /
router.get('/', function(req, res, next) {
return res.render('index', { title: 'Home' });
});
// GET /about
router.get('/about', function(req, res, next) {
return res.render('about', { title: 'About' });
});
// GET /contact
router.get('/contact', function(req, res, next) {
return res.render('contact', { title: 'Contact' });
});
module.exports = router;
1 Answer

Alexander La Bianca
15,959 PointsI am havving troubble reading your code. Please format it with
javascript
However, I am looking at your post route for register where you post the user data. I see you do User.create(userData).
Where are you creating that userData? from what I see now it is undefined which could be why there is nothing being saved.
Ivan Penchev
13,833 PointsIvan Penchev
13,833 PointsPlease format your code properly.