Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial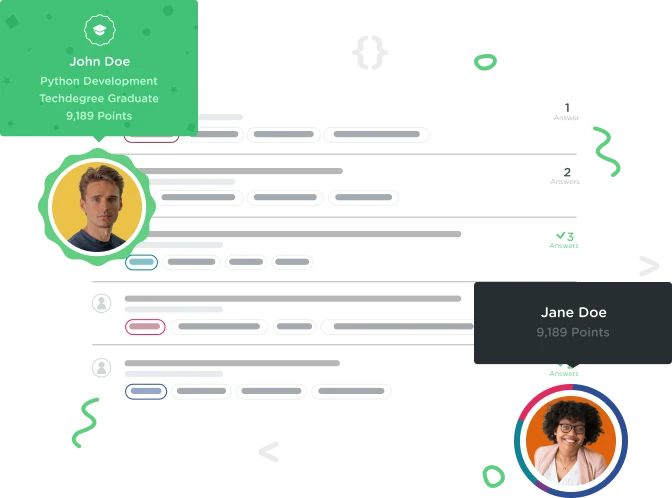

Carney Bernard
3,615 PointsI don't know why this code isn't right...
I keep getting a ton of errors. The initial question was confusing as is already:
"Since a quantity of 1 is such a common argument when adding a product to the cart, your fellow developers have asked you to make the following code work, as well as keeping the ability to add a product and a quantity."
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
public void addItem (Product addedItem) {
addedItem(addItem, 1);
}
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
5 Answers

Ken Alger
Treehouse TeacherCarney;
You are having errors with the syntax in your ShoppingCart.java
file.
What we need to do is to create an additional method that will utilize the same syntax as before, but just automatically add a quantity of "1" when the object is created. As it stands right now during the object creation the quantity must be specified, correct?
How would we then go about that? Our addItem
method currently is:
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
In order to create a similar method that can be used interchangeably, we need to set it up the same way as a separate method, therefore the code snippet would look similar to:
public void addItem(Product item, int quantity) {
// current code
}
public void addItem(Product item) {
// magical code to meet the challenge
}
So, what do we put inside our "Magical code block" that will help? We already have what we are looking to do in our first addItem
method, correct? So we should be able to have the new method, which takes different parameters and therefore Java is able to differentiate between them, utilize our old method and simply pass in our required parameter of a quantity of "1", make sense? We could then make our new addItem
method look like:
public void addItem(Product item) {
addItem(item, 1);
}
Now in our shopping cart app, in Example.java
we can create new objects with a quantity of 1 simply by cart.addItem("Darth Vader Dispenser")
or cart.addItem("Jar Jar Binks")
. At the same time if we wanted to order multiples of something, we could do cart.addItem("Princess Leia", 5)
and our application would know how to handle all of those statements.
I hope that helped more than it confused.
Happy coding,
Ken
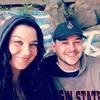
John Orduno
15,848 PointsWithout giving away too much:
The biggest problem I've seen is how the challenge is worded. The terminology is a bit confusing, but after reading all of these answers, I can understand it better. Rob's response to Ivan clears that up a bit.
Use a method signature = Use a method of the same name but with a different number of parameters. This was covered in an earlier lesson, but like most people probably reading this, I forgot all about it when posed with the challenge lol.
In this case, you need to make a method of the same name as addItem(), but it only passes on the item name. The quantity needs to be automatically set to 1.
The program, then, needs to be able to call that method only using the product name parameter (the code that needs to be un-commented in the example.java class needs to be able to work when you un-comment it. addItem(dispenser); ) Also, the previous calling of the method needs to work.... addItem(pez,5);. This requires the original method to remain intact.
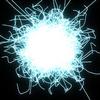
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHello, a problem that I can see is in your shopping cart class. The new method is written correctly, however you wrote it inside the first method, so the system gets confused. Try to make it look something like this
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
public void addItem (Product addedItem) {
addItem(addedItem, 1);
}
That should help, let me know if it doesn't.

Carney Bernard
3,615 PointsRob thanks for your help! It did not work but eventually I figured it out!

Ivan Yudhi
2,031 PointsI want to try doing this problem without help, but I still don't understand what I'm supposed to do.
/* Since a quantity of 1 is such a common argument when adding a product to the cart, * your fellow developers have asked you to make the following code work, as well as keeping * the ability to add a product and a quantity. */
Okay, what following code? This one?
Product dispenser = new Product("Yoda PEZ dispenser");
// Uncomment this line after using method signatures to solve their request in ShoppingCart.java
// cart.addItem(dispenser);
}
I guess I should look at addItem method on ShoppingCart.java
/* Other code omitted for clarity */
Well, not much help here..
Can you improve the instructions please? What exactly am I suppose to do here?
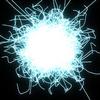
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey Ivan,
I'll see if I can help out with this.
The first thing that Craig is wanting you to do is set create a brand new method called drive, you don't need to delete or modify the existing method at all. When you're in the challenge this code is actually in ShoppingCart class.
When creating your new drive method, be sure to call it the same thing, because of something called method signatures in Java there's a difference between these two methods even if they are called the same thing, as long as they take different parameters.
In the previous method that is already created two arguments need to be passed into it, an item from the Product class, and an amount.
In the new method we are creating, have your drive method just accept an item from the Product class, much like the previous method, however with this method do not make the method require that a quantity be passed into it like the other method, this method should just accept a single argument, and that is an item from the Product class, much like the method above it.
One you create this new method, have the body of the method use the already defined method previously that accepted two arguments, just ensure that when they use your new method, the default quantity is always one.
Thanks, let me know if this doesn't help and I'll try to explain it a bit better.

Jess Sanders
12,086 PointsExample.java has a line of code that is commented out
cart.addItem(dispenser);
If all you do is uncomment this line and check your work, you get a compiler error. We need to write a second addItem method that only takes one argument, rather than two.
So, in ShoppingCart.java, we can copy and paste the existing addItem method, but remove the second argument. We also need to change what is printed, because we know that we are Adding 1 of the item to the cart.
Carney Bernard
3,615 PointsCarney Bernard
3,615 PointsThank you for you help Ken! your step by step breakdown definitely helped! Typically when you tackle challenges and experience problems when you are programming what is your approach? I'm trying to get better at solving these challenges without the aid of others. Thanks again!
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherCarney;
I really try to simplify things down to as much of a step-by-step process as possible. Andrew Chalkley has a course jQuery Basics and goes over a great thought process for development and he carries it out to varying degrees in his other courses as well. I don't believe it is original to him, but he calls it the 4 P's of Problem Solving. Preparation, Plan, Perform, and Perfect. It involves basically exactly what it sounds like.
How does this apply to Treehouse challenges? It is my opinion that most of the challenges are not designed to out and out trick us or to make us come up with a solution based on knowledge not covered in a particular course. For example, in the Java Basics and Objects courses there are undoubtedly other ways to achieve the same end results that have not been taught yet in those courses. Therefore, we aren't expected to know them for the challenges.
We can, therefore, reasonably assume that to prepare for a particular challenge, it should be sufficient to have completed the course up to that point. The plan is often laid out in the instructions or code comments. The perform is where we often get stuck in the challenges.
I think this step comes mostly with practice. That being said I know folks that have been coding for decades that still forget closing brackets, end of line semi-colons, have typos in variable names, etc. By in large those errors are corrected through lots of practice looking at code and being a good proof-reader.
The perfecting portion can at times be subjective. If the challenge is to output "Hello World" to the screen, there really is only so much we can do, right? With the challenges we don't need to implicitly worry about perfecting the code, if it passes we are happy. In the real world, perfecting can encompass refactoring, adding/subtracting features, etc.
Just my thoughts.
Ken
Carney Bernard
3,615 PointsCarney Bernard
3,615 PointsThanks for your comments Ken! I definitely appreciate that well thought out response! Completely agree...
Chris Batt
11,610 PointsChris Batt
11,610 PointsThat challenge escalated quickly. Thanks for clearing that up and also for answering about your technique above. The problem wasn't that hard after you broke it all down, but it seemed really (almost too) complicated at first.
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherChris;
Method overloading can be a challenge to grasp, but is an important concept. I think that while some of the courses here at Treehouse have challenges that are very similar to the video(s) and corresponding code, if not exactly the same, there seems to be a trend towards taking the knowledge and topics covered in the videos and making them so they cover the same knowledge base, but we aren't simply regurgitating the same code. I find this to be a useful technique for effective learning, perhaps others disagree, but being able to understand the concept and implement it in a slightly different context is, I think, powerful learning.
Just my thoughts.
Ken
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsI just kind of randomly found this amazing answer and subsequent comment section. This makes me wish the forums had a curated section, so that great discourse like this can be easily found.