Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial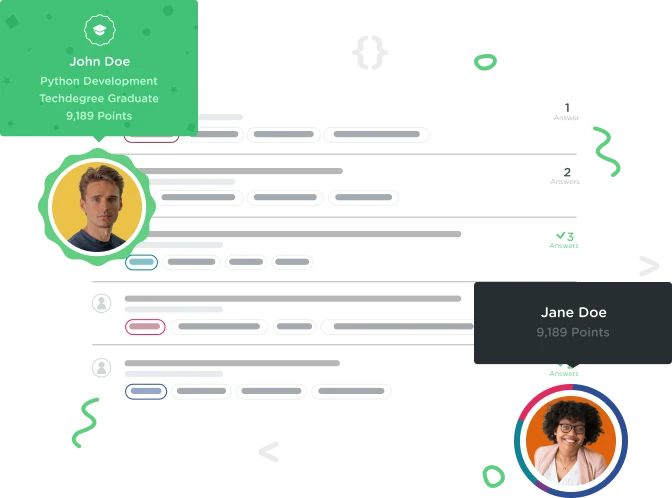

Matthew Musni
610 PointsI don't much get how to properly execute the catch Format exception
my program seems to respond when there's an invalid input but i dont know how to start over back to top to reset and ask input from the user
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int number = 0;
string input = Console.ReadLine();
while (true)
{
try
{
var final = int.Parse(input);
Console.WriteLine("Yay!");
number += 1;
if (final == number)
{
Console.ReadKey();
break;
}
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
Console.ReadKey();
}
continue;
}
}
}
}

Matthew Musni
610 Pointsyeah i want to ask for input again

Bernadette Isaacson
13,736 PointsOne way to do it, would be to use TryParse:
static void Main(string[] args)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string result = Console.ReadLine();
int x;
while (!int.TryParse(result, out x))
{
Console.WriteLine("You must enter a whole number.");
Console.Write("Enter the number of times to print \"Yay!\": ");
result = Console.ReadLine();
}
for (int i = 1; i <= x; i++)
{
Console.WriteLine("Yay!");
}
}
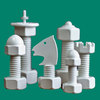
Steven Parker
229,732 PointsWhile tryParse can be used for validity testing in your own code, this challenge specifically requires try and catch.
You do not want to ask for input again, that will fail the challenge.
1 Answer
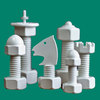
Steven Parker
229,732 PointsI see a few issues here:
- if the input is bad, the loop in the current code runs forever
- you might want to test the number just once, before the loop
- you don't want to call ReadKey inside (or after) the loop, it would keep the program from ending
- an explicit continue is unnecessary at the end of a loop
Jon Wood
9,884 PointsJon Wood
9,884 PointsIt looks like it does repeat when I input something invalid. Did you want it to ask for input again after it does that?