Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial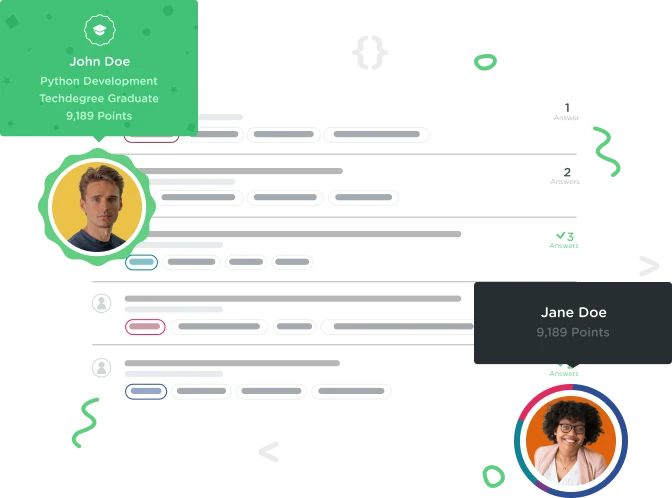
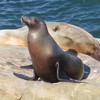
otarie
984 PointsI don't quite understand the way enumerate works so I'm having trouble solving this challenge. Can someone please help?
i don't understand the concept of enumerate
# combo(['swallow', 'snake', 'parrot'], 'abc')
# Output:
# [('swallow', 'a'), ('snake', 'b'), ('parrot', 'c')]
# If you use list.append(), you'll want to pass it a tuple of new values.
# Using enumerate() here can save you a variable or two.
3 Answers
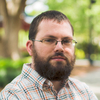
Kenneth Love
Treehouse Guest Teacherenumerate()
takes an iterable and gives back a tuple. That tuple has two items in it. The first item is the current index. So the first time through, it's 0. The next time, it's 1. The second item in the tuple is whatever is in the iterable at that index. Let's say we're going through the alphabet. The first time through, enumerate()
would give back (0, 'a')
. We'd get (1, 'b')
the second time. And so on.
So if we have two iterables of the same length, and enumerate()
gives us the items from one of them, how would you use the index that enumerate()
gives us to find the items in the second iterable?
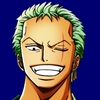
David Sudermann
Courses Plus Student 3,228 PointsI guess I cheated a little on that one by using the zip-function:
def combo(iterable_1, iterable_2):
return list(zip(iterable_1, iterable_2))
A simple way to do it with enumeration is this:
def combo(iterable_1, iterable_2):
tuple_list = []
for item in enumerate(iterable_1):
tuple_list.append((item[1], iterable_2[item[0]]))
return tuple_list
But you can do it without the use of any variables using enumeration as well:
def combo(iterable_1, iterable_2):
return [tuple for tuple in [(item[1], iterable_2[item[0]]) for item in enumerate(iterable_1)]]
Note:
Only the the first combo()-function works with iterables of uneven length without further adjustments!

lfkwtz
12,295 PointsMine was similar:
def combo(iterable_1,iterable_2):
blank = list()
for index, word in enumerate(iterable_1):
blank.append((word, iterable_2[index]))
return blank
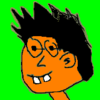
otarie
984 Pointsotarie
984 PointsThis is the challenge: Create a function named combo() that takes two iterables and returns a list of tuples. Each tuple should hold the first item in each list, then the second set, then the third, and so on. Assume the iterables will be the same length.