Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial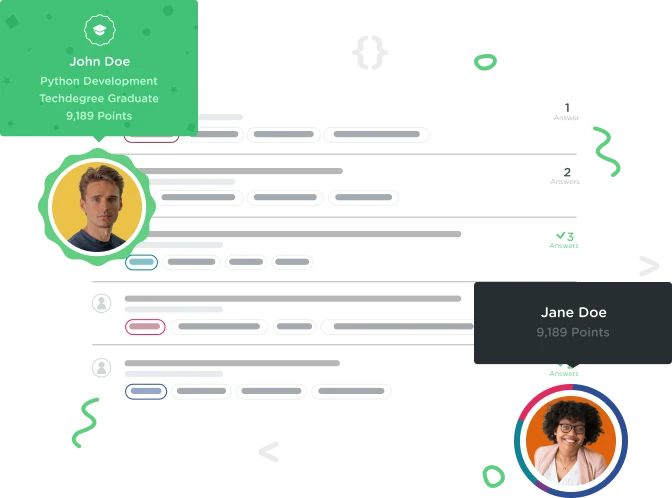

Carel Du Plessis
Courses Plus Student 16,356 PointsI don't quite understand why my code doesn't display values.
I initialized a List with the bird object as the data type so that I can go through it and display the values by using the foreach loop.
the code that doesn't work I have commented out.
// Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Ex_LINQ
{
class Program
{
static void Main(string[] args)
{
// This doesn't work
//List<Bird> birds = new List<Bird>();
//foreach (Bird bird in birds)
//{
//Console.WriteLine(bird.Sightings);
// }
//This works???
List<Bird> birds = BirdRepository.LoadBirds();
foreach (Bird bird in birds){
Console.WriteLine();
Console.WriteLine("Bird Name: " + bird.Name);
Console.WriteLine("Bird Color: " + bird.Color);
Console.WriteLine("Bird Sightings: " + bird.Sightings);
}
Console.ReadKey();
}
}
}
// Bird.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Ex_LINQ
{
public class Bird
{
public string Name { get; set; }
public string Color { get; set; }
public int Sightings { get; set; }
}
}
// BirdRepository.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Ex_LINQ
{
public static class BirdRepository
{
public static List<Bird> LoadBirds()
{
//Refactor the LoadBirds method by using object initialization to add the pelican to the birds variable.
List<Bird> birds = new List<Bird>{
new Bird {Name = "Pelican", Color = "White", Sightings = 3}
};
return birds;
}
}
}
2 Answers

Dominic Powell
4,051 PointsFor the commented code, you have a list of birds that you have initialized but have no value. So Console.WriteLine has nothing to write since the bird.Sightings property is empty. look more at your BirdRepository class

Kiron Roy
3,210 PointsI took elements of the code an came up with this is works. It can be also be checkout here
using System;
using System.Collections.Generic;
using System.Linq;
namespace LINQStuff
{
class Program
{
static void Main()
{
var birds = new List<Bird>
{
new Bird { Name = "Cardinal", Color = "Red", Sightings = 3 },
new Bird { Name = "Dove", Color = "White", Sightings = 2 } ,
new Bird { Name = "Robin", Color = "Red", Sightings = 5 } ,
new Bird { Name = "Blue Jay", Color = "Blue", Sightings = 1 } ,
};
var canary = new Bird { Name = "Canary", Color = "Yellow", Sightings = 0 };
birds.Add(canary);
foreach (Bird bird in birds)
{
Console.WriteLine();
Console.WriteLine($" Bird Name: {bird.Name} \n " +
$"Bird Color: {bird.Color} \n " +
$"Bird Sightings: {bird.Sightings} " );
}
Console.ReadLine(); // this takes out the line "press any key to continue"
}
}
}